Comparisons
We know many comparison operators from maths.
In JavaScript they are written like this:
- Greater/less than: a > b , a < b .
- Greater/less than or equals: a >= b , a <= b .
- Equals: a == b , please note the double equality sign == means the equality test, while a single one a = b means an assignment.
- Not equals: In maths the notation is ≠ , but in JavaScript it’s written as a != b .
In this article we’ll learn more about different types of comparisons, how JavaScript makes them, including important peculiarities.
At the end you’ll find a good recipe to avoid “JavaScript quirks”-related issues.

Boolean is the result
All comparison operators return a boolean value:
- true – means “yes”, “correct” or “the truth”.
- false – means “no”, “wrong” or “not the truth”.
For example:
A comparison result can be assigned to a variable, just like any value:
String comparison
To see whether a string is greater than another, JavaScript uses the so-called “dictionary” or “lexicographical” order.
In other words, strings are compared letter-by-letter.
The algorithm to compare two strings is simple:
- Compare the first character of both strings.
- If the first character from the first string is greater (or less) than the other string’s, then the first string is greater (or less) than the second. We’re done.
- Otherwise, if both strings’ first characters are the same, compare the second characters the same way.
- Repeat until the end of either string.
- If both strings end at the same length, then they are equal. Otherwise, the longer string is greater.
In the first example above, the comparison 'Z' > 'A' gets to a result at the first step.
The second comparison 'Glow' and 'Glee' needs more steps as strings are compared character-by-character:
- G is the same as G .
- l is the same as l .
- o is greater than e . Stop here. The first string is greater.
The comparison algorithm given above is roughly equivalent to the one used in dictionaries or phone books, but it’s not exactly the same.
For instance, case matters. A capital letter "A" is not equal to the lowercase "a" . Which one is greater? The lowercase "a" . Why? Because the lowercase character has a greater index in the internal encoding table JavaScript uses (Unicode). We’ll get back to specific details and consequences of this in the chapter Strings .
Comparison of different types
When comparing values of different types, JavaScript converts the values to numbers.
For boolean values, true becomes 1 and false becomes 0 .
It is possible that at the same time:
- Two values are equal.
- One of them is true as a boolean and the other one is false as a boolean.
From JavaScript’s standpoint, this result is quite normal. An equality check converts values using the numeric conversion (hence "0" becomes 0 ), while the explicit Boolean conversion uses another set of rules.
Strict equality
A regular equality check == has a problem. It cannot differentiate 0 from false :
The same thing happens with an empty string:
This happens because operands of different types are converted to numbers by the equality operator == . An empty string, just like false , becomes a zero.
What to do if we’d like to differentiate 0 from false ?
A strict equality operator === checks the equality without type conversion.
In other words, if a and b are of different types, then a === b immediately returns false without an attempt to convert them.
Let’s try it:
There is also a “strict non-equality” operator !== analogous to != .
The strict equality operator is a bit longer to write, but makes it obvious what’s going on and leaves less room for errors.
Comparison with null and undefined
There’s a non-intuitive behavior when null or undefined are compared to other values.
These values are different, because each of them is a different type.
There’s a special rule. These two are a “sweet couple”: they equal each other (in the sense of == ), but not any other value.
null/undefined are converted to numbers: null becomes 0 , while undefined becomes NaN .
Now let’s see some funny things that happen when we apply these rules. And, what’s more important, how to not fall into a trap with them.
Strange result: null vs 0
Let’s compare null with a zero:
Mathematically, that’s strange. The last result states that " null is greater than or equal to zero", so in one of the comparisons above it must be true , but they are both false.
The reason is that an equality check == and comparisons > < >= <= work differently. Comparisons convert null to a number, treating it as 0 . That’s why (3) null >= 0 is true and (1) null > 0 is false.
On the other hand, the equality check == for undefined and null is defined such that, without any conversions, they equal each other and don’t equal anything else. That’s why (2) null == 0 is false.
An incomparable undefined
The value undefined shouldn’t be compared to other values:
Why does it dislike zero so much? Always false!
We get these results because:
- Comparisons (1) and (2) return false because undefined gets converted to NaN and NaN is a special numeric value which returns false for all comparisons.
- The equality check (3) returns false because undefined only equals null , undefined , and no other value.
Avoid problems
Why did we go over these examples? Should we remember these peculiarities all the time? Well, not really. Actually, these tricky things will gradually become familiar over time, but there’s a solid way to avoid problems with them:
- Treat any comparison with undefined/null except the strict equality === with exceptional care.
- Don’t use comparisons >= > < <= with a variable which may be null/undefined , unless you’re really sure of what you’re doing. If a variable can have these values, check for them separately.
- Comparison operators return a boolean value.
- Strings are compared letter-by-letter in the “dictionary” order.
- When values of different types are compared, they get converted to numbers (with the exclusion of a strict equality check).
- The values null and undefined equal == each other and do not equal any other value.
- Be careful when using comparisons like > or < with variables that can occasionally be null/undefined . Checking for null/undefined separately is a good idea.
What will be the result for these expressions?
Some of the reasons:
- Obviously, true.
- Dictionary comparison, hence false. "a" is smaller than "p" .
- Again, dictionary comparison, first char "2" is greater than the first char "1" .
- Values null and undefined equal each other only.
- Strict equality is strict. Different types from both sides lead to false.
- Similar to (4) , null only equals undefined .
- Strict equality of different types.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
- Español – América Latina
- Português – Brasil
- Tiếng Việt
Comparison operators
Comparison operators compare the values of two operands and evaluate whether the statement they form is true or false . The following example uses the strict equality operator ( === ) to compare two operands: the expression 2 + 2 and the value 4 . Because the result of the expression and the number value 4 are the same, this expression evaluates to true :
Type coercion and equality
Two of the most frequently-used comparison operators are == for loose equality and === for strict equality. == performs a loose comparison between two values by coercing the operands to matching data types, if possible. For example, 2 == "2" returns true , even though the comparison is being made between a number value and a string value.
The same is true of != , which returns true only if the operands being compared aren't loosely equal.
Strict comparisons using === or !== don't perform type coercion. For a strict comparison to evaluate to true , the values being compared must have the same data type. Because of this, 2 == "2" returns true , but 2 === "2" returns false :
To remove any ambiguity that might result from auto-coercion, use === whenever possible.
Operator | Description | Usage | Result |
---|---|---|---|
=== | Strictly equal | 2 === 2 | true |
!== | Not strictly-equal | 2 !== "2" | true |
== | Equal (or "loosely equal") | 2 == "2" | true |
!= | Not equal | 2 != "3" | true |
> | Greater than | 3 > 2 | true |
>= | Greater than or equal to | 2 >= 2 | true |
< | Less than | 2 < 3 | true |
<= | Less than or equal to | 2 <= 3 | true |
Truthy and falsy
All values in JavaScript are implicitly true or false , and can be coerced to the corresponding boolean value—for example, by using the "loosely equal" comparator. A limited set of values coerce to false :
- An empty string ( "" )
All other values coerce to true , including any string containing one or more characters and all nonzero numbers. These are commonly called "truthy" and "falsy" values.
Logical operators
Use the logical AND ( && ), OR ( || ), and NOT ( ! ) operators to control the flow of a script based on the evaluation of two or more conditional statements:
A logical NOT ( ! ) expression negates the truthy or falsy value of an operand, evaluating to true if the operand evaluates to false , and false if the operand evaluates to true :
Using the logical NOT operator ( ! ) in front of another data type, like a number or a string, coerces that value to a boolean and reverses the truthy or falsy value of the result.
It's common practice to use two NOT operators to quickly coerce data to its matching boolean value:
The logical AND and OR operators don't perform any coercion by themselves. They return the value of one of the two operands being evaluated, with the chosen operand determined by that evaluation.
Logical AND ( && ) returns the first of its two operands only if that operand evaluates to false , and the second operand otherwise. In comparisons that evaluate to boolean values, it returns true only if the operands on both sides of the logical AND evaluate to true . If either side evaluates to false , it returns false .
When && is used with two non-boolean operands, the first operand is returned unchanged if it can be coerced to false . If the first operand can be coerced to true , the second operand is returned unchanged:
Logical OR ( || ) returns the first of its two operands only if that operand evaluates to true , and the second operand otherwise. In comparisons that evaluate to boolean values, this means it returns true if either operand evaluates to true , and if neither side evaluates to true , it returns false :
When using || with two non-boolean operands, it returns the first operand unchanged if it could be coerced to true . If the first operand can be coerced to false , the second operand is returned unchanged:
Nullish coalescing operator
Introduced in ES2020 , the "nullish coalescing operator" ( ?? ) returns the first operand only if that operand has any value other than null or undefined . Otherwise, it returns the second operand.
?? is similar to a logical OR, but stricter in how the first operand is evaluated. || returns the second operand for any expression that can be coerced to false , including undefined and null . ?? returns the second operand when the first operand is anything but null or undefined , even if it could be coerced to false :
Logical assignment operators
Use assignment operators to assign the value of a second operator to a first operator. The most common example of this is a single equals sign ( = ), used to assign a value to a declared variable .
Use logical assignment operators to conditionally assign a value to a variable based on the truthy or falsy value of that variable.
The logical AND assignment ( &&= ) operator evaluates the second operand and assigns to the first operand if the only if the first operand would evaluate to true —effectively, "if the first operand is true, assign it the value of the second operand instead:"
The truthy or falsy value of the first operand determines whether an assignment is performed. However, trying to evaluate the first operand using a comparison operator results in a true or false boolean, which can't be assigned a value:
The logical OR assignment ( ||= ) operator evaluates the second operand and assign to the first operand if the first operand evaluates to false — effectively "if the first operand is false, assign it the value of the second operand instead:"
Check your understanding
Which operator indicates "strictly equal"?
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2024-03-31 UTC.
- Skip to main content
- Select language
- Skip to search
- Expressions and operators
- Operator precedence
Left-hand-side expressions
« Previous Next »
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more.
A complete and detailed list of operators and expressions is also available in the reference .
JavaScript has the following types of operators. This section describes the operators and contains information about operator precedence.
- Assignment operators
- Comparison operators
- Arithmetic operators
- Bitwise operators
Logical operators
String operators, conditional (ternary) operator.
- Comma operator
Unary operators
- Relational operator
JavaScript has both binary and unary operators, and one special ternary operator, the conditional operator. A binary operator requires two operands, one before the operator and one after the operator:
For example, 3+4 or x*y .
A unary operator requires a single operand, either before or after the operator:
For example, x++ or ++x .
An assignment operator assigns a value to its left operand based on the value of its right operand. The simple assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x .
There are also compound assignment operators that are shorthand for the operations listed in the following table:
Name | Shorthand operator | Meaning |
---|---|---|
Destructuring
For more complex assignments, the destructuring assignment syntax is a JavaScript expression that makes it possible to extract data from arrays or objects using a syntax that mirrors the construction of array and object literals.
A comparison operator compares its operands and returns a logical value based on whether the comparison is true. The operands can be numerical, string, logical, or object values. Strings are compared based on standard lexicographical ordering, using Unicode values. In most cases, if the two operands are not of the same type, JavaScript attempts to convert them to an appropriate type for the comparison. This behavior generally results in comparing the operands numerically. The sole exceptions to type conversion within comparisons involve the === and !== operators, which perform strict equality and inequality comparisons. These operators do not attempt to convert the operands to compatible types before checking equality. The following table describes the comparison operators in terms of this sample code:
Operator | Description | Examples returning true |
---|---|---|
( ) | Returns if the operands are equal. |
|
( ) | Returns if the operands are not equal. | |
( ) | Returns if the operands are equal and of the same type. See also and . | |
( ) | Returns if the operands are of the same type but not equal, or are of different type. | |
( ) | Returns if the left operand is greater than the right operand. | |
( ) | Returns if the left operand is greater than or equal to the right operand. | |
( ) | Returns if the left operand is less than the right operand. | |
( ) | Returns if the left operand is less than or equal to the right operand. |
Note: ( => ) is not an operator, but the notation for Arrow functions .
An arithmetic operator takes numerical values (either literals or variables) as their operands and returns a single numerical value. The standard arithmetic operators are addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). These operators work as they do in most other programming languages when used with floating point numbers (in particular, note that division by zero produces Infinity ). For example:
In addition to the standard arithmetic operations (+, -, * /), JavaScript provides the arithmetic operators listed in the following table:
Operator | Description | Example |
---|---|---|
( ) | Binary operator. Returns the integer remainder of dividing the two operands. | 12 % 5 returns 2. |
( ) | Unary operator. Adds one to its operand. If used as a prefix operator ( ), returns the value of its operand after adding one; if used as a postfix operator ( ), returns the value of its operand before adding one. | If is 3, then sets to 4 and returns 4, whereas returns 3 and, only then, sets to 4. |
( ) | Unary operator. Subtracts one from its operand. The return value is analogous to that for the increment operator. | If is 3, then sets to 2 and returns 2, whereas returns 3 and, only then, sets to 2. |
( ) | Unary operator. Returns the negation of its operand. | If is 3, then returns -3. |
( ) | Unary operator. Attempts to convert the operand to a number, if it is not already. | returns . returns |
( ) | Calculates the to the power, that is, | returns . returns . |
A bitwise operator treats their operands as a set of 32 bits (zeros and ones), rather than as decimal, hexadecimal, or octal numbers. For example, the decimal number nine has a binary representation of 1001. Bitwise operators perform their operations on such binary representations, but they return standard JavaScript numerical values.
The following table summarizes JavaScript's bitwise operators.
Operator | Usage | Description |
---|---|---|
Returns a one in each bit position for which the corresponding bits of both operands are ones. | ||
Returns a zero in each bit position for which the corresponding bits of both operands are zeros. | ||
Returns a zero in each bit position for which the corresponding bits are the same. [Returns a one in each bit position for which the corresponding bits are different.] | ||
Inverts the bits of its operand. | ||
Shifts in binary representation bits to the left, shifting in zeros from the right. | ||
Shifts in binary representation bits to the right, discarding bits shifted off. | ||
Shifts in binary representation bits to the right, discarding bits shifted off, and shifting in zeros from the left. |
Bitwise logical operators
Conceptually, the bitwise logical operators work as follows:
- The operands are converted to thirty-two-bit integers and expressed by a series of bits (zeros and ones). Numbers with more than 32 bits get their most significant bits discarded. For example, the following integer with more than 32 bits will be converted to a 32 bit integer: Before: 11100110111110100000000000000110000000000001 After: 10100000000000000110000000000001
- Each bit in the first operand is paired with the corresponding bit in the second operand: first bit to first bit, second bit to second bit, and so on.
- The operator is applied to each pair of bits, and the result is constructed bitwise.
For example, the binary representation of nine is 1001, and the binary representation of fifteen is 1111. So, when the bitwise operators are applied to these values, the results are as follows:
Expression | Result | Binary Description |
---|---|---|
Note that all 32 bits are inverted using the Bitwise NOT operator, and that values with the most significant (left-most) bit set to 1 represent negative numbers (two's-complement representation).
Bitwise shift operators
The bitwise shift operators take two operands: the first is a quantity to be shifted, and the second specifies the number of bit positions by which the first operand is to be shifted. The direction of the shift operation is controlled by the operator used.
Shift operators convert their operands to thirty-two-bit integers and return a result of the same type as the left operand.
The shift operators are listed in the following table.
Operator | Description | Example |
---|---|---|
( ) | This operator shifts the first operand the specified number of bits to the left. Excess bits shifted off to the left are discarded. Zero bits are shifted in from the right. | yields 36, because 1001 shifted 2 bits to the left becomes 100100, which is 36. |
( ) | This operator shifts the first operand the specified number of bits to the right. Excess bits shifted off to the right are discarded. Copies of the leftmost bit are shifted in from the left. | yields 2, because 1001 shifted 2 bits to the right becomes 10, which is 2. Likewise, yields -3, because the sign is preserved. |
( ) | This operator shifts the first operand the specified number of bits to the right. Excess bits shifted off to the right are discarded. Zero bits are shifted in from the left. | yields 4, because 10011 shifted 2 bits to the right becomes 100, which is 4. For non-negative numbers, zero-fill right shift and sign-propagating right shift yield the same result. |
Logical operators are typically used with Boolean (logical) values; when they are, they return a Boolean value. However, the && and || operators actually return the value of one of the specified operands, so if these operators are used with non-Boolean values, they may return a non-Boolean value. The logical operators are described in the following table.
Operator | Usage | Description |
---|---|---|
( ) | Returns if it can be converted to ; otherwise, returns . Thus, when used with Boolean values, returns if both operands are true; otherwise, returns . | |
( ) | Returns if it can be converted to ; otherwise, returns . Thus, when used with Boolean values, returns if either operand is true; if both are false, returns . | |
( ) | Returns if its single operand can be converted to ; otherwise, returns . |
Examples of expressions that can be converted to false are those that evaluate to null, 0, NaN, the empty string (""), or undefined.
The following code shows examples of the && (logical AND) operator.
The following code shows examples of the || (logical OR) operator.
The following code shows examples of the ! (logical NOT) operator.
Short-circuit evaluation
As logical expressions are evaluated left to right, they are tested for possible "short-circuit" evaluation using the following rules:
- false && anything is short-circuit evaluated to false.
- true || anything is short-circuit evaluated to true.
The rules of logic guarantee that these evaluations are always correct. Note that the anything part of the above expressions is not evaluated, so any side effects of doing so do not take effect.
In addition to the comparison operators, which can be used on string values, the concatenation operator (+) concatenates two string values together, returning another string that is the union of the two operand strings.
For example,
The shorthand assignment operator += can also be used to concatenate strings.
The conditional operator is the only JavaScript operator that takes three operands. The operator can have one of two values based on a condition. The syntax is:
If condition is true, the operator has the value of val1 . Otherwise it has the value of val2 . You can use the conditional operator anywhere you would use a standard operator.
This statement assigns the value "adult" to the variable status if age is eighteen or more. Otherwise, it assigns the value "minor" to status .
The comma operator ( , ) simply evaluates both of its operands and returns the value of the last operand. This operator is primarily used inside a for loop, to allow multiple variables to be updated each time through the loop.
For example, if a is a 2-dimensional array with 10 elements on a side, the following code uses the comma operator to update two variables at once. The code prints the values of the diagonal elements in the array:
A unary operation is an operation with only one operand.
The delete operator deletes an object, an object's property, or an element at a specified index in an array. The syntax is:
where objectName is the name of an object, property is an existing property, and index is an integer representing the location of an element in an array.
The fourth form is legal only within a with statement, to delete a property from an object.
You can use the delete operator to delete variables declared implicitly but not those declared with the var statement.
If the delete operator succeeds, it sets the property or element to undefined . The delete operator returns true if the operation is possible; it returns false if the operation is not possible.
Deleting array elements
When you delete an array element, the array length is not affected. For example, if you delete a[3] , a[4] is still a[4] and a[3] is undefined.
When the delete operator removes an array element, that element is no longer in the array. In the following example, trees[3] is removed with delete . However, trees[3] is still addressable and returns undefined .
If you want an array element to exist but have an undefined value, use the undefined keyword instead of the delete operator. In the following example, trees[3] is assigned the value undefined , but the array element still exists:
The typeof operator is used in either of the following ways:
The typeof operator returns a string indicating the type of the unevaluated operand. operand is the string, variable, keyword, or object for which the type is to be returned. The parentheses are optional.
Suppose you define the following variables:
The typeof operator returns the following results for these variables:
For the keywords true and null , the typeof operator returns the following results:
For a number or string, the typeof operator returns the following results:
For property values, the typeof operator returns the type of value the property contains:
For methods and functions, the typeof operator returns results as follows:
For predefined objects, the typeof operator returns results as follows:
The void operator is used in either of the following ways:
The void operator specifies an expression to be evaluated without returning a value. expression is a JavaScript expression to evaluate. The parentheses surrounding the expression are optional, but it is good style to use them.
You can use the void operator to specify an expression as a hypertext link. The expression is evaluated but is not loaded in place of the current document.
The following code creates a hypertext link that does nothing when the user clicks it. When the user clicks the link, void(0) evaluates to undefined , which has no effect in JavaScript.
The following code creates a hypertext link that submits a form when the user clicks it.
Relational operators
A relational operator compares its operands and returns a Boolean value based on whether the comparison is true.
The in operator returns true if the specified property is in the specified object. The syntax is:
where propNameOrNumber is a string or numeric expression representing a property name or array index, and objectName is the name of an object.
The following examples show some uses of the in operator.
The instanceof operator returns true if the specified object is of the specified object type. The syntax is:
where objectName is the name of the object to compare to objectType , and objectType is an object type, such as Date or Array .
Use instanceof when you need to confirm the type of an object at runtime. For example, when catching exceptions, you can branch to different exception-handling code depending on the type of exception thrown.
For example, the following code uses instanceof to determine whether theDay is a Date object. Because theDay is a Date object, the statements in the if statement execute.
The precedence of operators determines the order they are applied when evaluating an expression. You can override operator precedence by using parentheses.
The following table describes the precedence of operators, from highest to lowest.
Operator type | Individual operators |
---|---|
member | |
call / create instance | |
negation/increment | |
multiply/divide | |
addition/subtraction | |
bitwise shift | |
relational | |
equality | |
bitwise-and | |
bitwise-xor | |
bitwise-or | |
logical-and | |
logical-or | |
conditional | |
assignment | |
comma |
A more detailed version of this table, complete with links to additional details about each operator, may be found in JavaScript Reference .
- Expressions
An expression is any valid unit of code that resolves to a value.
Every syntactically valid expression resolves to some value but conceptually, there are two types of expressions: with side effects (for example: those that assign value to a variable) and those that in some sense evaluates and therefore resolves to value.
The expression x = 7 is an example of the first type. This expression uses the = operator to assign the value seven to the variable x . The expression itself evaluates to seven.
The code 3 + 4 is an example of the second expression type. This expression uses the + operator to add three and four together without assigning the result, seven, to a variable. JavaScript has the following expression categories:
- Arithmetic: evaluates to a number, for example 3.14159. (Generally uses arithmetic operators .)
- String: evaluates to a character string, for example, "Fred" or "234". (Generally uses string operators .)
- Logical: evaluates to true or false. (Often involves logical operators .)
- Primary expressions: Basic keywords and general expressions in JavaScript.
- Left-hand-side expressions: Left values are the destination of an assignment.
Primary expressions
Basic keywords and general expressions in JavaScript.
Use the this keyword to refer to the current object. In general, this refers to the calling object in a method. Use this either with the dot or the bracket notation:
Suppose a function called validate validates an object's value property, given the object and the high and low values:
You could call validate in each form element's onChange event handler, using this to pass it the form element, as in the following example:
- Grouping operator
The grouping operator ( ) controls the precedence of evaluation in expressions. For example, you can override multiplication and division first, then addition and subtraction to evaluate addition first.
Comprehensions
Comprehensions are an experimental JavaScript feature, targeted to be included in a future ECMAScript version. There are two versions of comprehensions:
Comprehensions exist in many programming languages and allow you to quickly assemble a new array based on an existing one, for example.
Left values are the destination of an assignment.
You can use the new operator to create an instance of a user-defined object type or of one of the built-in object types. Use new as follows:
The super keyword is used to call functions on an object's parent. It is useful with classes to call the parent constructor, for example.
Spread operator
The spread operator allows an expression to be expanded in places where multiple arguments (for function calls) or multiple elements (for array literals) are expected.
Example: Today if you have an array and want to create a new array with the existing one being part of it, the array literal syntax is no longer sufficient and you have to fall back to imperative code, using a combination of push , splice , concat , etc. With spread syntax this becomes much more succinct:
Similarly, the spread operator works with function calls:
Document Tags and Contributors
- l10n:priority
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Legacy generator function expression
- Logical Operators
- Object initializer
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript Comparison and Logical Operators
JavaScript Ternary Operator
JavaScript Booleans
JavaScript Bitwise Operators
- JavaScript Object.is()
- JavaScript typeof Operator
JavaScript operators are special symbols that perform operations on one or more operands (values). For example,
Here, we used the + operator to add the operands 2 and 3 .
JavaScript Operator Types
Here is a list of different JavaScript operators you will learn in this tutorial:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- String Operators
- Miscellaneous Operators
1. JavaScript Arithmetic Operators
We use arithmetic operators to perform arithmetic calculations like addition, subtraction, etc. For example,
Here, we used the - operator to subtract 3 from 5 .
Commonly Used Arithmetic Operators
Operator | Name | Example |
---|---|---|
Addition | ||
Subtraction | ||
Multiplication | ||
Division | ||
Remainder | ||
Increment (increments by ) | or | |
Decrement (decrements by ) | or | |
Exponentiation (Power) |
Example 1: Arithmetic Operators in JavaScript
Note: The increment operator ++ adds 1 to the operand. And, the decrement operator -- decreases the value of the operand by 1 .
To learn more, visit Increment ++ and Decrement -- Operators .
2. JavaScript Assignment Operators
We use assignment operators to assign values to variables. For example,
Here, we used the = operator to assign the value 5 to the variable x .
Commonly Used Assignment Operators
Operator | Name | Example |
---|---|---|
Assignment Operator | ||
Addition Assignment | ||
Subtraction Assignment | ||
Multiplication Assignment | ||
Division Assignment | ||
Remainder Assignment | ||
Exponentiation Assignment |
Example 2: Assignment Operators in JavaScript
3. javascript comparison operators.
We use comparison operators to compare two values and return a boolean value ( true or false ). For example,
Here, we have used the > comparison operator to check whether a (whose value is 3 ) is greater than b (whose value is 2 ).
Since 3 is greater than 2 , we get true as output.
Note: In the above example, a > b is called a boolean expression since evaluating it results in a boolean value.
Commonly Used Comparison Operators
Operator | Meaning | Example |
---|---|---|
Equal to | gives us | |
Not equal to | gives us | |
Greater than | gives us | |
Less than | gives us | |
Greater than or equal to | gives us | |
Less than or equal to | gives us | |
Strictly equal to | gives us | |
Strictly not equal to | gives us |
Example 3: Comparison Operators in JavaScript
The equality operators ( == and != ) convert both operands to the same type before comparing their values. For example,
Here, we used the == operator to compare the number 3 and the string 3 .
By default, JavaScript converts string 3 to number 3 and compares the values.
However, the strict equality operators ( === and !== ) do not convert operand types before comparing their values. For example,
Here, JavaScript didn't convert string 4 to number 4 before comparing their values.
Thus, the result is false , as number 4 isn't equal to string 4 .
4. JavaScript Logical Operators
We use logical operators to perform logical operations on boolean expressions. For example,
Here, && is the logical operator AND . Since both x < 6 and y < 5 are true , the combined result is true .
Commonly Used Logical Operators
Operator | Syntax | Description |
---|---|---|
(Logical AND) | only if both and are | |
(Logical OR) | if either or is | |
(Logical NOT) | if is and vice versa |
Example 4: Logical Operators in JavaScript
Note: We use comparison and logical operators in decision-making and loops. You will learn about them in detail in later tutorials.
More on JavaScript Operators
We use bitwise operators to perform binary operations on integers.
Operator | Description | Example |
---|---|---|
& | Bitwise AND | |
| | Bitwise OR | |
^ | Bitwise XOR | |
~ | Bitwise NOT | |
<< | Left shift | |
>> | Sign-propagating right shift | |
>>> | Zero-fill right shift |
Note: We rarely use bitwise operators in everyday programming. If you are interested, visit JavaScript Bitwise Operators to learn more.
In JavaScript, you can also use the + operator to concatenate (join) two strings. For example,
Here, we used the + operator to concatenate str1 and str2 .
JavaScript has many more operators besides the ones we listed above. You will learn about them in detail in later tutorials.
Operator | Description | Example |
---|---|---|
: Evaluates multiple operands and returns the value of the last operand. | ||
: Returns value based on the condition. | ||
Returns the data type of the variable. | ||
Returns t if the specified object is a valid object of the specified class. | ||
Discards any expression's return value. |
Table of Contents
- Introduction
- JavaScript Arithmetic Operators
- JavaScript Assignment Operators
- JavaScript Comparison Operators
- JavaScript Logical Operators
Video: JavaScript Operators
Sorry about that.
Related Tutorials
JavaScript Tutorial
Learn JavaScript Operators – Logical, Comparison, Ternary, and More JS Operators With Examples

JavaScript has many operators that you can use to perform operations on values and variables (also called operands)
Based on the types of operations these JS operators perform, we can divide them up into seven groups:
Arithmetic Operators
Assignment operators, comparison operators, logical operators.
- Ternary Operators
The typeof Operator
Bitwise operators.
In this handbook, you're going to learn how these operators work with examples. Let's start with arithmetic operators.
The arithmetic operators are used to perform mathematical operations like addition and subtraction.
These operators are frequently used with number data types, so they are similar to a calculator. The following example shows how you can use the + operator to add two variables together:
Here, the two variables x and y are added together using the plus + operator. We also used the console.log() method to print the result of the operation to the screen.
You can use operators directly on values without assigning them to any variable too:
In JavaScript, we have 8 arithmetic operators in total. They are:
- Subtraction -
- Multiplication *
- Remainder %
- Exponentiation **
- Increment ++
- Decrement --
Let's see how these operators work one by one.
1. Addition operator
The addition operator + is used to add two or more numbers together. You've seen how this operator works previously, but here's another example:
You can use the addition operator on both integer and floating numbers.
2. Subtraction operator
The subtraction operator is marked by the minus sign − and you can use it to subtract the right operand from the left operand.
For example, here's how to subtract 3 from 5:
3. Multiplication operator
The multiplication operator is marked by the asterisk * symbol, and you use it to multiply the value on the left by the value on the right of the operator.
4. Division operator
The division operator / is used to divide the left operand by the right operand. Here are some examples of using the operator:
5. Remainder operator
The remainder operator % is also known as the modulo or modulus operator. This operator is used to calculate the remainder after a division has been performed.
A practical example should make this operator easier to understand, so let's see one:
The number 10 can't be divided by 3 perfectly. The result of the division is 3 with a remainder of 1. The remainder operator simply returns that remainder number.
If the left operand can be divided with no remainder, then the operator returns 0.
This operator is commonly used when you want to check if a number is even or odd. If a number is even, dividing it by 2 will result in a remainder of 0, and if it's odd, the remainder will be 1.
6. Exponentiation operator
The exponentiation operator is marked by two asterisks ** . It's one of the newer JavaScript operators and you can use it to calculate the power of a number (based on its exponent).
For example, here's how to calculate 10 to the power of 3:
Here, the number 10 is multiplied by itself 3 times (10 _ 10 _ 10)
The exponentiation operator gives you an easy way to find the power of a specific number.
7. Increment operator
The increment ++ operator is used to increase the value of a number by one. For example:
This operator gives you a faster way to increase a variable value by one. Without the operator, here's how you increment a variable:
Using the increment operator allows you to shorten the second line. You can place this operator before or next to the variable you want to increment:
Both placements shown above are valid. The difference between prefix (before) and postfix (after) placements is that the prefix position will execute the operator after that line of code has been executed.
Consider the following example:
Here, you can see that placing the increment operator next to the variable will print the variable as if it has not been incremented.
When you place the operator before the variable, then the number will be incremented before calling the console.log() method.

8. Decrement operator
The decrement -- operator is used to decrease the value of a number by one. It's the opposite of the increment operator:
Please note that you can only use increment and decrement operators on a variable. An error occurs when you try to use these operators directly on a number value:
You can't use increment or decrement operator on a number directly.
Arithmetic operators summary
Now you've learned the 8 types of arithmetic operators. Excellent! Keep in mind that you can mix these operators to perform complex mathematical equations.
For example, you can perform an addition and multiplication on a set of numbers:
The order of operations in JavaScript is the same as in mathematics. Multiplication, division, and exponentiation take a higher priority than addition or subtraction (remember that acronym PEMDAS? Parentheses, exponents, multiplication and division, addition and subtraction – there's your order of operations).
You can use parentheses () to change the order of the operations. Wrap the operation you want to execute first as follows:
When using increment or decrement operators together with other operators, you need to place the operators in a prefix position as follows:
This is because a postfix increment or decrement operator will not be executed together with other operations in the same line, as I have explained previously.
Let's try some exercises. Can you guess the result of these operations?
And that's all for arithmetic operators. You've done a wonderful job learning about these operators.
Let's take a short five-minute break before proceeding to the next type of operators.
The second group of operators we're going to explore is the assignment operators.
Assignment operators are used to assign a specific value to a variable. The basic assignment operator is marked by the equal = symbol, and you've already seen this operator in action before:
After the basic assignment operator, there are 5 more assignment operators that combine mathematical operations with the assignment. These operators are useful to make your code clean and short.
For example, suppose you want to increment the x variable by 2. Here's how you do it with the basic assignment operator:
There's nothing wrong with the code above, but you can use the addition assignment += to rewrite the second line as follows:
There are 7 kinds of assignment operators that you can use in JavaScript:
Name | Operation example | Meaning |
---|---|---|
Assignment | ||
Addition assignment | ||
Subtraction assignment | ||
Multiplication assignment | ||
Division assignment | ||
Remainder assignment | ||
Exponentiation assignment |
The arithmetic operators you've learned in the previous section can be combined with the assignment operator except the increment and decrement operators.
Let's have a quick exercise. Can you guess the results of these assignments?
Now you've learned about assignment operators. Let's continue and learn about comparison operators.
As the name implies, comparison operators are used to compare one value or variable with something else. The operators in this category always return a boolean value: either true or false .
For example, suppose you want to compare if a variable's value is greater than 1. Here's how you do it:
The greater than > operator checks if the value on the left operand is greater than the value on the right operand.
There are 8 kinds of comparison operators available in JavaScript:
Name | Operation example | Meaning |
---|---|---|
Equal | Returns if the operands are equal | |
Not equal | Returns if the operands are not equal | |
Strict equal | Returns if the operands are equal and have the same type | |
Strict not equal | Returns if the operands are not equal, or have different types | |
Greater than | Returns if the left operand is greater than the right operand | |
Greater than or equal | Returns if the left operand is greater than or equal to the right operand | |
Less than | Returns if the left operand is less than the right operand | |
Less than or equal | Returns if the left operand is less than or equal to the right operand |
Here are some examples of using comparison operators:
The comparison operators are further divided in two types: relational and equality operators.
The relational operators compare the value of one operand relative to the second operand (greater than, less than)
The equality operators check if the value on the left is equal to the value on the right. They can also be used to compare strings like this:
String comparisons are case-sensitive, as shown in the example above.
JavaScript also has two versions of the equality operators: loose and strict.
In strict mode, JavaScript will compare the values without performing a type coercion. To enable strict mode, you need to add one more equal = symbol to the operation as follows:
Since type coercion might result in unwanted behavior, you should use the strict equality operators anytime you do an equality comparison.
Logical operators are used to check whether one or more expressions result in either true or false .
There are three logical operators available in JavaScript:
Name | Operation example | Meaning |
---|---|---|
Logical AND | Returns if all operands are , else returns | |
Logical OR | Returns if one of the operands is , else returns | |
Logical NOT | Reverse the result: returns if and vice versa |
These operators can only return Boolean values. For example, you can determine whether '7 is greater than 2' and '5 is greater than 4':
These logical operators follow the laws of mathematical logic:
- && AND operator – if any expression returns false , the result is false
- || OR operator – if any expression returns true , the result is true
- ! NOT operator – negates the expression, returning the opposite.
Let's do a little exercise. Try to run these statements on your computer. Can you guess the results?
These logical operators will come in handy when you need to assert that a specific requirement is fulfilled in your code.
Let's say a happyLife requires a job with highIncome and supportiveTeam :
Based on the requirements, you can use the logical AND operator to check whether you have both requirements. When one of the requirements is false , then happyLife equals false as well.
Ternary Operator
The ternary operator (also called the conditional operator) is the only JavaScipt operator that requires 3 operands to run.
Let's imagine you need to implement some specific logic in your code. Suppose you're opening a shop to sell fruit. You give a $3 discount when the total purchase is $20 or more. Otherwise, you give a $1 discount.
You can implement the logic using an if..else statement as follows:
The code above works fine, but you can use the ternary operator to make the code shorter and more concise as follows:
The syntax for the ternary operator is condition ? expression1 : expression2 .
You need to write the condition to evaluate followed by a question ? mark.
Next to the question mark, you write the expression to execute when the condition evaluates to true , followed by a colon : symbol. You can call this expression1 .
Next to the colon symbol, you write the expression to execute when the condition evaluates to false . This is expression2 .
As the example above shows, the ternary operator can be used as an alternative to the if..else statement.
The typeof operator is the only operator that's not represented by symbols. This operator is used to check the data type of the value you placed on the right side of the operator.
Here are some examples of using the operator:
The typeof operator returns the type of the data as a string. The 'number' type represents both integer and float types, the string and boolean represent their respective types.
Arrays, objects, and the null value are of object type, while undefined has its own type.
Bitwise operators are operators that treat their operands as a set of binary digits, but return the result of the operation as a decimal value.
These operators are rarely used in web development, so you can skip this part if you only want to learn practical stuff. But if you're interested to know how they work, then let me show you an example.
A computer uses a binary number system to store decimal numbers in memory. The binary system only uses two numbers, 0 and 1, to represent the whole range of decimal numbers we humans know.
For example, the decimal number 1 is represented as binary number 00000001, and the decimal number 2 is represented as 00000010.
I won't go into detail on how to convert a decimal number into a binary number as that's too much to include in this guide. The main point is that the bitwise operators operate on these binary numbers.
If you want to find the binary number from a specific decimal number, you can Google for the "decimal to binary calculator".
There are 7 types of bitwise operators in JavaScript:
- Left Shift <<
- Right Shift >>
- Zero-fill Right Shift >>>
Let's see how they work.
1. Bitwise AND operator
The bitwise operator AND & returns a 1 when the number 1 overlaps in both operands. The decimal numbers 1 and 2 have no overlapping 1, so using this operator on the numbers return 0:
2. Bitwise OR operator
On the other hand, the bitwise operator OR | returns all 1s in both decimal numbers.
The binary number 00000011 represents the decimal number 3, so the OR operator above returns 3.
Bitwise XOR operator
The Bitwise XOR ^ looks for the differences between two binary numbers. When the corresponding bits are the same, it returns 0:
5 = 00000101
Bitwise NOT operator
Bitwise NOT ~ operator inverts the bits of a decimal number so 0 becomes 1 and 1 becomes 0:
Bitwise Left Shift operator
Bitwise Left Shift << shifts the position of the bit by adding zeroes from the right.
The excess bits are then discarded, changing the decimal number represented by the bits. See the following example:
The right operand is the number of zeroes you will add to the left operand.
Bitwise Right Shift operator
Bitwise Right Shift >> shifts the position of the bits by adding zeroes from the left. It's the opposite of the Left Shift operator:
Bitwise Zero-fill Right Shift operator
Also known as Unsigned Right Shift operator, the Zero-fill Right Shift >>> operator is used to shift the position of the bits to the right, while also changing the sign bit to 0 .
This operator transforms any negative number into a positive number, so you can see how it works when passing a negative number as the left operand:
In the above example, you can see that the >> and >>> operators return different results. The Zero-fill Right Shift operator has no effect when you use it on a positive number.
Now you've learned how the bitwise operators work. If you think they are confusing, then you're not alone! Fortunately, these operators are scarcely used when developing web applications.
You don't need to learn them in depth. It's enough to know what they are.
In this tutorial, you've learned the 7 types of JavaScript operators: Arithmetic, assignment, comparison, logical, ternary, typeof, and bitwise operators.
These operators can be used to manipulate values and variables to achieve a desired outcome.
Congratulations on finishing this guide!
If you enjoyed this article and want to take your JavaScript skills to the next level, I recommend you check out my new book Beginning Modern JavaScript here .
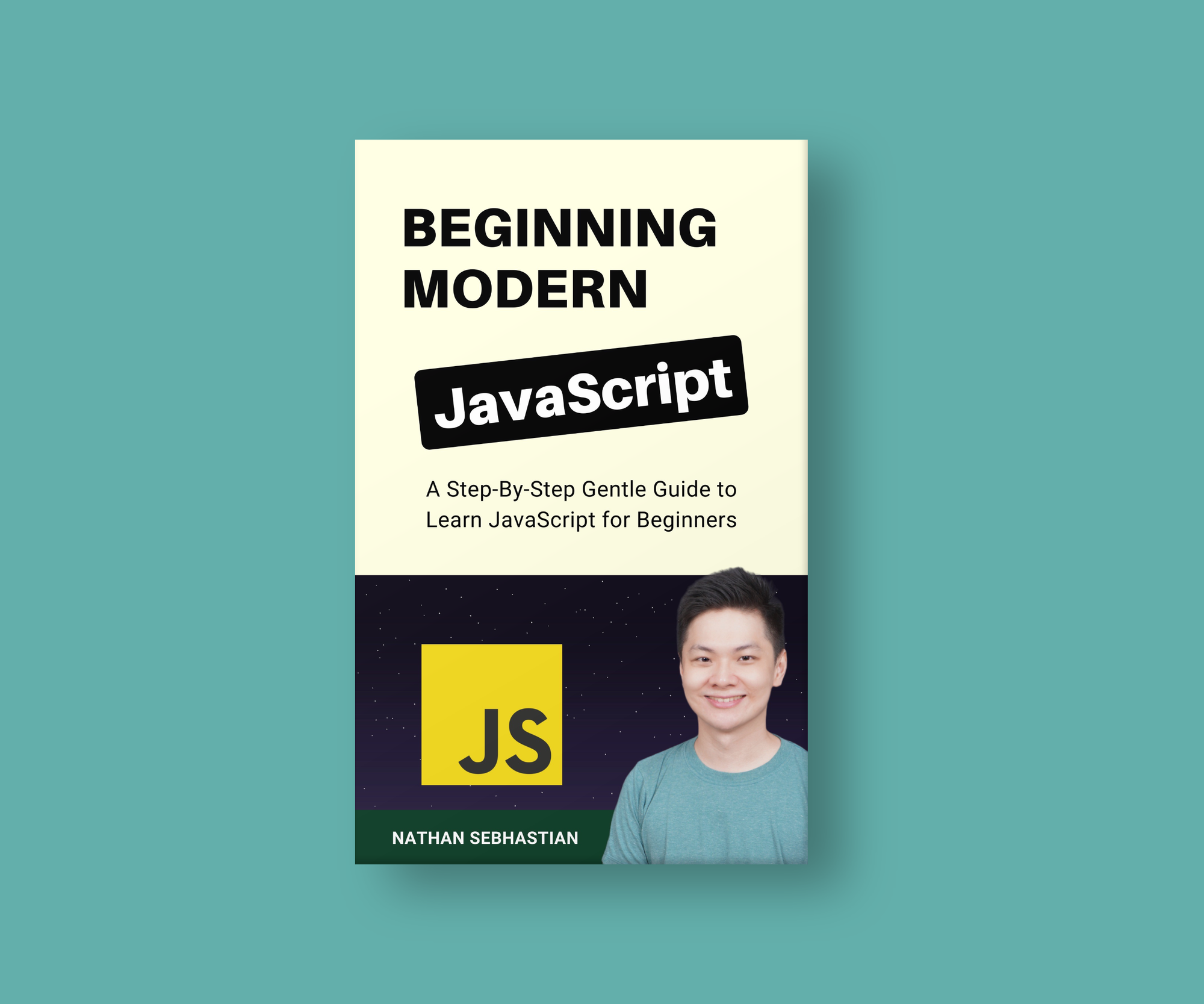
The book is designed to be easy to understand and accessible to anyone looking to learn JavaScript. It provides a step-by-step gentle guide that will help you understand how to use JavaScript to create a dynamic application.
Here's my promise: You will actually feel like you understand what you're doing with JavaScript.
Until next time!
JavaScript Full Stack Developer currently working with fullstack JS using React and Express. Nathan loves to write about his experience in programming to help other people.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Home » JavaScript Tutorial » An Introduction to JavaScript Logical Operators
An Introduction to JavaScript Logical Operators
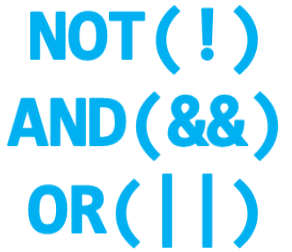
Summary : in this tutorial, you will learn how to use the JavaScript logical operators including the logical NOT operator( ! ), the logical AND operator ( && ) and the logical OR operator ( || ).
The logical operators are important in JavaScript because they allow you to compare variables and do something based on the result of that comparison.
For example, if the result of the comparison is true , you can run a block of code; if it’s false , you can execute another code block.
JavaScript provides three logical operators:
- ! (Logical NOT)
- || (Logical OR)
- && (Logical AND)
1) The Logical NOT operator (!)
JavaScript uses an exclamation point ! to represent the logical NOT operator. The ! operator can be applied to a single value of any type, not just a Boolean value.
When you apply the ! operator to a boolean value, the ! returns true if the value is false and vice versa. For example:
In this example, the eligible is true so !eligible returns false . And since the required is true , the !required returns false .
When you apply the ! operator to a non-Boolean value. The ! operator converts the value to a boolean value and then negates it.
The following example shows how to use the ! operator:
The logical ! operator works based on the following rules:
- If a is undefined , the result is true .
- If a is null , the result is true .
- If a is a number other than 0 , the result is false .
- If a is NaN , the result is true .
- If a is an object , the result is false.
- If a is an empty string, the result is true . If a is a non-empty string, the result is false
The following demonstrates the results of the logical ! operator when applying to a non-boolean value:
Double-negation ( !! )
Sometimes, you may see the double negation ( !! ) in the code. The !! uses the logical NOT operator ( ! ) twice to convert a value to its real boolean value.
The result is the same as using the Boolean() function. For example:
The first ! operator negates the Boolean value of the counter variable. If the counter is true , then the ! operator makes it false and vice versa.
The second ! operator negates the result of the first ! operator and returns the real boolean value of the counter variable.
2) The Logical AND operator (&&)
JavaScript uses the double ampersand ( && ) to represent the logical AND operator. The following expression uses the && operator:
If a can be converted to true , the && operator returns the b ; otherwise, it returns the a . This rule is applied to all boolean values.
The following truth table illustrates the result of the && operator when it is applied to two Boolean values:
a | b | a && b |
---|---|---|
true | true | true |
true | false | false |
false | true | false |
false | false | false |
The result of the && operator is true only if both values are true , otherwise, it is false . For example:
In this example, the eligible is false , therefore, the value of the expression eligible && required is false .
See the following example:
In this example, both eligible and required are true , therefore, the value of the expression eligible && required is true .
Short-circuit evaluation
The && operator is short-circuited. It means that the && operator evaluates the second value only if the first one doesn’t suffice to determine the value of an expression. For example:
In this example, b is true therefore the && operator could not determine the result without further evaluating the second expression ( 1/0 ).
The result is Infinity which is the result of the expression ( 1/0 ). However:
In this case, b is false , the && operator doesn’t need to evaluate the second expression because it can determine the final result as false based value of the first value.
The chain of && operators
The following expression uses multiple && operators:
The && operator carries the following:
- Evaluates values from left to right.
- For each value, convert it to a boolean. If the result is false , stops and returns the original value.
- If all values are truthy values, return the last value.
In other words, The && operator returns the first falsey value or the last truthy value.
If a value can be converted to true , it is called a truthy value. If a value can be converted to false , it is a called a falsey value.
3) The Logical OR operator (||)
JavaScript uses the double-pipe || to represent the logical OR operator. You can apply the || operator to two values of any type:
If a can be converted to true , returns a ; else, returns b . This rule is also applied to boolean values.
The following truth table illustrates the result of the || operator based on the value of the operands:
a | b | a || b |
---|---|---|
true | true | true |
true | false | true |
false | true | true |
false | false | false |
The || operator returns false if both values are evaluated to false . In case either value is true , the || operator returns true . For example:
See another example:
In this example, the expression eligible || required returns false because both values are false .
The || operator is also short-circuited
Similar to the && operator, the || operator is short-circuited. It means that if the first value evaluates to true , the && operator doesn’t evaluate the second one.
The chain of || operators
The following example shows how to use multiple || operators in an expression:
The || operator does the following:
- For each value, convert it to a boolean value. If the result of the conversion is true , stops and returns the value.
- If all values have been evaluated to false , returns the last value.
In other words, the chain of the || operators returns the first truthy value or the last one if no truthy value is found.
Logical operator precedence
When you mix logical operators in an expression, the JavaScript engine evaluates the operators based on a specified order. This order is called the operator precedence .
In other words, the operator precedence is the order of evaluating logical operators in an expression.
The precedence of the logical operator is in the following order from the highest to the lowest:
- Logical NOT (!)
- Logical AND (&&)
- Logical OR (||)
- The NOT operator ( ! ) negates a boolean value. The ( !! ) converts a value into its real boolean value.
- The AND operator ( && ) is applied to two Boolean values and returns true if both are true.
- The OR operator ( || ) is applied to two Boolean values and returns true if one of the operands is true .
- Both && and || operator are short-circuited. They can also be applied to non-Boolean values.
- The logical operator precedence from the highest to the lowest is ! , && and || .
- The AND operator returns the first falsey value or the last truthy value.
- The || operator returns the first falsey value.
- React Native
- CSS Frameworks
- JS Frameworks
- Web Development
JavaScript ‘===’ vs ‘==’Comparison Operator
In Javascript(ES6), there are four ways to test equality which are listed below:
- Using ‘==’ operator
- Using ‘===’ operator
- SameValueZero: used mainly in sets, maps and arrays.
- SameValue: used elsewhere
Now, our main concern is getting to know the difference between the ‘==’ and ‘===’ operators that the javascript provides, though they look similar, they are very different.
- JavaScript ‘==’ operator: In Javascript, the ‘==’ operator is also known as the loose equality operator which is mainly used to compare two values on both sides and then return true or false. This operator checks equality only after converting both the values to a common type i.e type coercion .
Note: Type coercion means that the two values are compared only after attempting to convert them into the same type. Let’s look at all the values in which the ‘==’ operator will return true.
Boolean values of literals during comparison:
- ‘0’
- ‘false’ // false wrapped in string.
- function(){}
- ” or “” // empty string
- NaN // not-a-number
Example 1:
Output: In the above code, when we compare 21 with ’21’ the javascript will convert the ’21’ into the number value of 21, and hence we get true, a similar thing happens when we try to check if ‘true == 1’, in that case, the javascript basically converts 1 into a truthy value and then compares and return the boolean true.
The case of (null == undefined) is special as when we compare these values we get true. Both null and undefined are false values and they represent an ’empty’ value or undefined in js, hence the comparison with ‘==’ operator returns true.
Now, let’s look at all the values in which the ‘==’ operator will return false.
Example 2: In this code when we compare null with false we get false, as null being a primitive data type it can never be equal to a boolean value, even though they belong to the same false group.
JavaScript ‘===’ operator: Also known as strict equality operator, it compares both the value and the type which is why the name “strict equality”.
Example 1: Let’s see some code where ‘===’ operator will return true.
Example 2: Simply check the types and values at both sides and then just print out the boolean true or false. Some examples where it will return false.
Please go through the Difference between double equal vs triple equal JavaScript article to compare ‘===’ and ‘==’ operators.
Please Login to comment...
Similar reads.
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript assignment, javascript assignment operators.
Assignment operators assign values to JavaScript variables.
Operator | Example | Same As |
---|---|---|
= | x = y | x = y |
+= | x += y | x = x + y |
-= | x -= y | x = x - y |
*= | x *= y | x = x * y |
/= | x /= y | x = x / y |
%= | x %= y | x = x % y |
**= | x **= y | x = x ** y |
Shift Assignment Operators
Operator | Example | Same As |
---|---|---|
<<= | x <<= y | x = x << y |
>>= | x >>= y | x = x >> y |
>>>= | x >>>= y | x = x >>> y |
Bitwise Assignment Operators
Operator | Example | Same As |
---|---|---|
&= | x &= y | x = x & y |
^= | x ^= y | x = x ^ y |
|= | x |= y | x = x | y |
Logical Assignment Operators
Operator | Example | Same As |
---|---|---|
&&= | x &&= y | x = x && (x = y) |
||= | x ||= y | x = x || (x = y) |
??= | x ??= y | x = x ?? (x = y) |
The = Operator
The Simple Assignment Operator assigns a value to a variable.
Simple Assignment Examples
The += operator.
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
The -= operator.
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
The *= operator.
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
The **= operator.
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
The /= operator.
The Division Assignment Operator divides a variable.
Division Assignment Example
The %= operator.
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
Advertisement
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
The >>= operator.
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
The >>>= operator.
The Unsigned Right Shift Assignment Operator right shifts a variable (unsigned).
Unsigned Right Shift Assignment Example
The &= operator.
The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
Bitwise AND Assignment Example
The |= operator.
The Bitwise OR Assignment Operator does a bitwise OR operation on two operands and assigns the result to the variable.
Bitwise OR Assignment Example
The ^= operator.
The Bitwise XOR Assignment Operator does a bitwise XOR operation on two operands and assigns the result to the variable.
Bitwise XOR Assignment Example
The &&= operator.
The Logical AND assignment operator is used between two values.
If the first value is true, the second value is assigned.
Logical AND Assignment Example
The &&= operator is an ES2020 feature .
The ||= Operator
The Logical OR assignment operator is used between two values.
If the first value is false, the second value is assigned.
Logical OR Assignment Example
The ||= operator is an ES2020 feature .
The ??= Operator
The Nullish coalescing assignment operator is used between two values.
If the first value is undefined or null, the second value is assigned.
Nullish Coalescing Assignment Example
The ??= operator is an ES2020 feature .
Test Yourself With Exercises
Use the correct assignment operator that will result in x being 15 (same as x = x + y ).
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Comparison Operator Vs. Assignment Operator In JavaScript
This is a piece of code from a simple Bookmarker App I made. I am kind of confused about something here. Look at the 3rd line of the code. Isn't there supposed to be == instead of = after classname ? Because = is an assignment operator. What I need is true which == or === should give and it indeed does from the console.log .
However when I use === inside the if statement the function no longer works. But it works with the = which is not making any sense to me. It would be great if someone could clarify what's the problem here.
If anyone would like to check the full code including the HTML and CSS, here it is: https://github.com/magnetickode/Bookmarking-App

- asignment operator always returns true in an if statement ... why the others do not work is not clear from this fragment of code you provided – Michel Engelen Commented Feb 18, 2018 at 9:50
- what is e.target.className? – xianshenglu Commented Feb 18, 2018 at 9:50
- 1 @MichelEngelen, no, if you assign a falsy value, then it returns the falsy value for the check. – Nina Scholz Commented Feb 18, 2018 at 9:52
- @xianshenglu ... I wanted to ask this as well ... it seems as if he is selecting by one class and adds an eventlistener depending on this ... so from my point of view the e.target.className of each of these elements should be at least bookmarks delete and therefore wpould never be true by checking only for one of the classNames – Michel Engelen Commented Feb 18, 2018 at 9:53
- @NinaScholz ok, you are right ... I should have been more clear with this one, thanks for the clarification! – Michel Engelen Commented Feb 18, 2018 at 9:54
2 Answers 2
change this:

- I already did that before. Getting functionality is not the problem. I'm confused about why className === 'delete' doesn't work when it should. – Magnetic Kode Commented Feb 18, 2018 at 9:58
- @MagneticKode, console.log(e.target.className === 'delete'); is this true or false?and what is the output of e.target.className – xianshenglu Commented Feb 18, 2018 at 10:01
- @MagneticKode,for what i have seen,your code:class="btn delete" means e.target.className==="btn delete" not "delete",is it ? – xianshenglu Commented Feb 18, 2018 at 10:04
- @MagneticKode,I see your button with class="btn delete",so e.target.className != 'delete' – xianshenglu Commented Feb 18, 2018 at 10:32
- Lol damn can't believe I didn't notice that. Thanks for pointing it out. It's all cleared now. – Magnetic Kode Commented Feb 18, 2018 at 10:34
Inside if statement we should use anything, which converts to boolean , so, as you mentioned, simple assignment doesn't make sense, because it always returns true . e.target.className contains all classes of the element, so you can't just do e.target.className == 'delete' or e.target.className === 'delete' if there is more than one class, because strings will not be equal ( "q w e" includes "q" , but "q" isn't equal to "q w e" ). I see, you're using ES6, so you can do e.target.className.includes('delete') , it checks, does the element contain class delete , (for more info about includes method for strings: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/includes ). It is also possible to use String.indexOf like element.className.indexOf('class') !== -1 to test for the existence of a class (or === -1 to test for it's absence).
And here is the example:
const bookmarks = document.querySelectorAll('.bookmarks'); for (let i = 0; i < bookmarks.length; i++) { bookmarks[i].addEventListener('click', deleteBookmark); } function deleteBookmark(e) { alert("Target contains 'delete' class? " + e.target.className.includes('delete').toString().toUpperCase()); /*if (e.target.className.includes('delete')) { e.target.parentNode.parentNode.removeChild(e.target.parentNode); for (let i = 0; i < bookmarks.length; i++) { if (bookmarks[i].name ===e.target.parentNode.getElementsByTagName('p')[0].textContent) { bookmarks.splice(i, 1); break; } } }*/ } <div class="bookmarks delete">CLICK (contains "delete" class)</div> <div class="bookmarks">CLICK (doesn't contain "delete" class)</div>
- The includes method does work. But I still don't get why className == doesn't work although there is only one class on the e.target I'm aiming for. – Magnetic Kode Commented Feb 18, 2018 at 10:26
- @MagneticKode, not really, there are at leas two, because you use document.querySelector('.bookmarks') (it's the first class), and then you check for delete class existence (it's the second). – P.S. Commented Feb 18, 2018 at 10:30
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged javascript or ask your own question .
- The Overflow Blog
- Community Products Roadmap Update, July 2024
- Featured on Meta
- We spent a sprint addressing your requests — here’s how it went
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- Policy: Generative AI (e.g., ChatGPT) is banned
- The [lib] tag is being burninated
- What makes a homepage useful for logged-in users
Hot Network Questions
- Mathematical expression of controlled Ry gate
- Concrete works by Alexandre Grothendieck, other than Dessin d'Enfants?
- Avoid underset text overlaping
- Solving complex opamp circuits
- Why does `p` not put all yanked lines when copying across files?
- confidence interval and rejection
- Are all Starship/Super Heavy "cylinders" 4mm thick?
- Sitting on a desk or at a desk? What's the diffrence?
- How does the temperature of the condenser impact an air conditioner's energy usage?
- Why does the voltage double at the end of line in a open transmission line (physical explanation)
- What is the best translation of the phrase "Live a nice life"?
- Rear disc brakes work intermittently
- Hourly pay rate calculation between Recruiting and Payroll Systems
- How to manage talkover in meetings?
- Why does Paul's fight with Feyd-Rautha take so long?
- What is the translation of "a discrete GPU" in French?
- How to group elements in order to use them like one character
- confidence intervals for proportions containing a theoretically impossible value (zero)
- Seeing edges where there are no edges
- How shall I find the device of a phone's storage so that I can mount it in Linux?
- Can the US president kill at will?
- If a lambda is declared as a default argument, is it different for each call site?
- When Canadian citizen residing abroad comes to visit Canada
- GDPR Data Processor
This is a potential security issue, you are being redirected to https://csrc.nist.gov .
You have JavaScript disabled. This site requires JavaScript to be enabled for complete site functionality.
An official website of the United States government
Here’s how you know
Official websites use .gov A .gov website belongs to an official government organization in the United States.
Secure .gov websites use HTTPS A lock ( Lock Locked padlock icon ) or https:// means you’ve safely connected to the .gov website. Share sensitive information only on official, secure websites.
Journal Article
Deep reinforcement learning-based task assignment for cooperative mobile edge computing.
Documentation Topics
Published: April 25, 2023 Citation: IEEE Transactions on Mobile Computing vol. 23, no. 4, (April 2024) pp. 3156-3171
Li-Tse Hsieh (Catholic University of America) , Hang Liu (Catholic University of America) , Yang Guo (NIST) , Robert Gazda (InterDigital, Inc.)
Mobile edge computing (MEC) integrates computing resources in wireless access networks to process computational tasks in close proximity to mobile users with low latency. This paper investigates the task assignment problem for cooperative MEC networks in which a set of geographically distributed heterogeneous edge servers not only cooperate with remote cloud data centers but also help each other to jointly process user tasks. We introduce a novel stochastic MEC cooperation framework to model the edge-to-edge horizontal cooperation and the edge-to-cloud vertical cooperation. The task assignment optimization problem is formulated by taking into consideration dynamic network states, uncertain node computing capabilities and task arrivals, as well as the heterogeneity of the involved entities. We then develop and compare three task assignment algorithms, based on different deep reinforcement learning (DRL) approaches, value-based, policy-based, and hybrid approaches. In addition, to reduce the search space and computation complexity of the algorithms, we propose decomposition and function approximation techniques by leveraging the structure of the underlying problem. The evaluation results show that the proposed DRL-based task assignment schemes outperform the existing algorithms, and the hybrid actor-critic scheme performs the best under dynamic MEC network environments.
Control Families
None selected
Documentation
Publication: https://doi.org/10.1109/TMC.2023.3270242 Preprint (pdf)
Supplemental Material: None available
Document History: 04/25/23: Journal Article (Final)
cloud & virtualization , mobile
communications & wireless

IMAGES
VIDEO
COMMENTS
When comparing a string with a number, JavaScript will convert the string to a number when doing the comparison. An empty string converts to 0. A non-numeric string converts to NaN which is always false. When comparing two strings, "2" will be greater than "12", because (alphabetically) 1 is less than 2.
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that ...
JavaScript comparison operators are essential tools for checking conditions and making decisions in your code. They are used to evaluate whether a condition is true or false by comparing variables or values. These operators play a crucial role in logical expressions, helping to determine the equality or differences between values.
Comparisons. We know many comparison operators from maths. In JavaScript they are written like this: Greater/less than: a > b, a < b. Greater/less than or equals: a >= b, a <= b. Equals: a == b, please note the double equality sign == means the equality test, while a single one a = b means an assignment.
Javascript operators are used to perform different types of mathematical and logical computations. Examples: The Assignment Operator = assigns values. The Addition Operator + adds values. The Multiplication Operator * multiplies values. The Comparison Operator > compares values
Comparison operators compare the values of two operands and evaluate whether the statement they form is true or false.The following example uses the strict equality operator (===) to compare two operands: the expression 2 + 2 and the value 4.Because the result of the expression and the number value 4 are the same, this expression evaluates to true:. 2 + 2 === 4 > true
The JavaScript comparison operators are as follows: Equals (==) Not Equal (!=) Strict Equal (===) Strict Not Equal (!==) Greater than (>) Greater than or equal (>=) ... Assignment Operators. Based on the value of its right operand, an assignment operator assigns a value to its left operand. The most fundamental application is the use of the ...
A comparison operator returns a Boolean value indicating whether the comparison is true or not. See the following example: let r1 = 20 > 10; // true let r2 = 20 < 10; // false let r3 = 10 == 10; // true Code language: JavaScript (javascript) A comparison operator takes two values. If the types of values are not comparable, the comparison ...
JavaScript Comparison Operators. Comparison operators compare two values and return a boolean value ( true or false ). For example, const a = 3, b = 2; console.log(a > b); // Output: true. Run Code. Here, we have used the > comparison operator to check whether a (whose value is 3) is greater than b (whose value is 2 ).
Expressions and operators. This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. A complete and detailed list of operators and expressions is also available in the reference.
People often compare double equals and triple equals by saying one is an "enhanced" version of the other. For example, double equals could be said as an extended version of triple equals, because the former does everything that the latter does, but with type conversion on its operands — for example, 6 == "6".Alternatively, it can be claimed that double equals is the baseline, and triple ...
JavaScript Assignment Operators. We use assignment operators to assign values to variables. For example, let x = 5; ... JavaScript Comparison Operators. We use comparison operators to compare two values and return a boolean value (true or false). For example,
= is the assignment operator. It sets a variable (the left-hand side) to a value (the right-hand side). The result is the value on the right-hand side. == is the comparison operator. It will only return true if both values are equivalent after coercing their types to the same type. === is a more strict comparison operator often called the ...
Conclusion. In this tutorial, you've learned the 7 types of JavaScript operators: Arithmetic, assignment, comparison, logical, ternary, typeof, and bitwise operators. These operators can be used to manipulate values and variables to achieve a desired outcome. Congratulations on finishing this guide!
Description. Logical AND assignment short-circuits, meaning that x &&= y is equivalent to x && (x = y), except that the expression x is only evaluated once. No assignment is performed if the left-hand side is not truthy, due to short-circuiting of the logical AND operator. For example, the following does not throw an error, despite x being const:
The following example shows how to use the ! operator: !a. The logical ! operator works based on the following rules: If a is undefined, the result is true. If a is null, the result is true. If a is a number other than 0, the result is false. If a is NaN, the result is true. If a is an object, the result is false.
JavaScript '==' operator: In Javascript, the '==' operator is also known as the loose equality operator which is mainly used to compare two values on both sides and then return true or false. This operator checks equality only after converting both the values to a common type i.e type coercion.
Basic keywords and general expressions in JavaScript. These expressions have the highest precedence (higher than operators ). The this keyword refers to a special property of an execution context. Basic null, boolean, number, and string literals. Array initializer/literal syntax. Object initializer/literal syntax.
Use the correct assignment operator that will result in x being 15 (same as x = x + y ). Start the Exercise. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
JavaScript comparison operators: Identity vs. Equality. 1. Javascript if-else operators why does whats the difference in checking for not equal. 1. Multiple Comparison / Assignment Operators on the Same Line in JavaScript. 36. Variable assignment inside an 'if' condition in JavaScript. 0.
We then develop and compare three task assignment algorithms, based on different deep reinforcement learning (DRL) approaches, value-based, policy-based, and hybrid approaches. In addition, to reduce the search space and computation complexity of the algorithms, we propose decomposition and function approximation techniques by leveraging the ...
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...