- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- Python Operators
- Precedence and Associativity of Operators in Python
- Python Arithmetic Operators
- Difference between / vs. // operator in Python
- Python - Star or Asterisk operator ( * )
- What does the Double Star operator mean in Python?
- Division Operators in Python
- Modulo operator (%) in Python
- Python Logical Operators
- Python OR Operator
- Difference between 'and' and '&' in Python
- not Operator in Python | Boolean Logic
- Ternary Operator in Python
- Python Bitwise Operators

Python Assignment Operators
Assignment operators in python.
- Walrus Operator in Python 3.8
- Increment += and Decrement -= Assignment Operators in Python
- Merging and Updating Dictionary Operators in Python 3.9
- New '=' Operator in Python3.8 f-string
Python Relational Operators
- Comparison Operators in Python
- Python NOT EQUAL operator
- Difference between == and is operator in Python
- Chaining comparison operators in Python
- Python Membership and Identity Operators
- Difference between != and is not operator in Python
The Python Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, and bitwise computations. The value the operator operates on is known as the Operand. Here, we will cover Different Assignment operators in Python .
Operators |
| ||
---|---|---|---|
| = | Assign the value of the right side of the expression to the left side operand | c = a + b |
| += | Add right side operand with left side operand and then assign the result to left operand | a += b |
| -= | Subtract right side operand from left side operand and then assign the result to left operand | a -= b |
| *= | Multiply right operand with left operand and then assign the result to the left operand | a *= b |
| /= | Divide left operand with right operand and then assign the result to the left operand | a /= b |
| %= | Divides the left operand with the right operand and then assign the remainder to the left operand | a %= b |
| //= | Divide left operand with right operand and then assign the value(floor) to left operand | a //= b |
| **= | Calculate exponent(raise power) value using operands and then assign the result to left operand | a **= b |
| &= | Performs Bitwise AND on operands and assign the result to left operand | a &= b |
| |= | Performs Bitwise OR on operands and assign the value to left operand | a |= b |
| ^= | Performs Bitwise XOR on operands and assign the value to left operand | a ^= b |
| >>= | Performs Bitwise right shift on operands and assign the result to left operand | a >>= b |
| <<= | Performs Bitwise left shift on operands and assign the result to left operand | a <<= b |
| := | Assign a value to a variable within an expression | a := exp |
Here are the Assignment Operators in Python with examples.
Assignment Operator
Assignment Operators are used to assign values to variables. This operator is used to assign the value of the right side of the expression to the left side operand.
Addition Assignment Operator
The Addition Assignment Operator is used to add the right-hand side operand with the left-hand side operand and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the addition assignment operator which will first perform the addition operation and then assign the result to the variable on the left-hand side.
S ubtraction Assignment Operator
The Subtraction Assignment Operator is used to subtract the right-hand side operand from the left-hand side operand and then assigning the result to the left-hand side operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the subtraction assignment operator which will first perform the subtraction operation and then assign the result to the variable on the left-hand side.
M ultiplication Assignment Operator
The Multiplication Assignment Operator is used to multiply the right-hand side operand with the left-hand side operand and then assigning the result to the left-hand side operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the multiplication assignment operator which will first perform the multiplication operation and then assign the result to the variable on the left-hand side.
D ivision Assignment Operator
The Division Assignment Operator is used to divide the left-hand side operand with the right-hand side operand and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the division assignment operator which will first perform the division operation and then assign the result to the variable on the left-hand side.
M odulus Assignment Operator
The Modulus Assignment Operator is used to take the modulus, that is, it first divides the operands and then takes the remainder and assigns it to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the modulus assignment operator which will first perform the modulus operation and then assign the result to the variable on the left-hand side.
F loor Division Assignment Operator
The Floor Division Assignment Operator is used to divide the left operand with the right operand and then assigs the result(floor value) to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the floor division assignment operator which will first perform the floor division operation and then assign the result to the variable on the left-hand side.
Exponentiation Assignment Operator
The Exponentiation Assignment Operator is used to calculate the exponent(raise power) value using operands and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the exponentiation assignment operator which will first perform exponent operation and then assign the result to the variable on the left-hand side.
Bitwise AND Assignment Operator
The Bitwise AND Assignment Operator is used to perform Bitwise AND operation on both operands and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise AND assignment operator which will first perform Bitwise AND operation and then assign the result to the variable on the left-hand side.
Bitwise OR Assignment Operator
The Bitwise OR Assignment Operator is used to perform Bitwise OR operation on the operands and then assigning result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise OR assignment operator which will first perform bitwise OR operation and then assign the result to the variable on the left-hand side.
Bitwise XOR Assignment Operator
The Bitwise XOR Assignment Operator is used to perform Bitwise XOR operation on the operands and then assigning result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise XOR assignment operator which will first perform bitwise XOR operation and then assign the result to the variable on the left-hand side.
Bitwise Right Shift Assignment Operator
The Bitwise Right Shift Assignment Operator is used to perform Bitwise Right Shift Operation on the operands and then assign result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise right shift assignment operator which will first perform bitwise right shift operation and then assign the result to the variable on the left-hand side.
Bitwise Left Shift Assignment Operator
The Bitwise Left Shift Assignment Operator is used to perform Bitwise Left Shift Opertator on the operands and then assign result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise left shift assignment operator which will first perform bitwise left shift operation and then assign the result to the variable on the left-hand side.
Walrus Operator
The Walrus Operator in Python is a new assignment operator which is introduced in Python version 3.8 and higher. This operator is used to assign a value to a variable within an expression.
Example: In this code, we have a Python list of integers. We have used Python Walrus assignment operator within the Python while loop . The operator will solve the expression on the right-hand side and assign the value to the left-hand side operand ‘x’ and then execute the remaining code.
Please Login to comment...
Similar reads.
- Python-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Certificates
Html and css, data analytics.
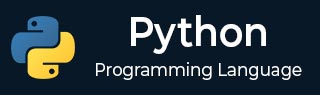
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Python Assignment Operator
The = (equal to) symbol is defined as assignment operator in Python. The value of Python expression on its right is assigned to a single variable on its left. The = symbol as in programming in general (and Python in particular) should not be confused with its usage in Mathematics, where it states that the expressions on the either side of the symbol are equal.
Example of Assignment Operator in Python
Consider following Python statements −
At the first instance, at least for somebody new to programming but who knows maths, the statement "a=a+b" looks strange. How could a be equal to "a+b"? However, it needs to be reemphasized that the = symbol is an assignment operator here and not used to show the equality of LHS and RHS.
Because it is an assignment, the expression on right evaluates to 15, the value is assigned to a.
In the statement "a+=b", the two operators "+" and "=" can be combined in a "+=" operator. It is called as add and assign operator. In a single statement, it performs addition of two operands "a" and "b", and result is assigned to operand on left, i.e., "a".
Augmented Assignment Operators in Python
In addition to the simple assignment operator, Python provides few more assignment operators for advanced use. They are called cumulative or augmented assignment operators. In this chapter, we shall learn to use augmented assignment operators defined in Python.
Python has the augmented assignment operators for all arithmetic and comparison operators.
Python augmented assignment operators combines addition and assignment in one statement. Since Python supports mixed arithmetic, the two operands may be of different types. However, the type of left operand changes to the operand of on right, if it is wider.
The += operator is an augmented operator. It is also called cumulative addition operator, as it adds "b" in "a" and assigns the result back to a variable.
The following are the augmented assignment operators in Python:
- Augmented Addition Operator
- Augmented Subtraction Operator
- Augmented Multiplication Operator
- Augmented Division Operator
- Augmented Modulus Operator
- Augmented Exponent Operator
- Augmented Floor division Operator
Augmented Addition Operator (+=)
Following examples will help in understanding how the "+=" operator works −
It will produce the following output −
Augmented Subtraction Operator (-=)
Use -= symbol to perform subtract and assign operations in a single statement. The "a-=b" statement performs "a=a-b" assignment. Operands may be of any number type. Python performs implicit type casting on the object which is narrower in size.
Augmented Multiplication Operator (*=)
The "*=" operator works on similar principle. "a*=b" performs multiply and assign operations, and is equivalent to "a=a*b". In case of augmented multiplication of two complex numbers, the rule of multiplication as discussed in the previous chapter is applicable.
Augmented Division Operator (/=)
The combination symbol "/=" acts as divide and assignment operator, hence "a/=b" is equivalent to "a=a/b". The division operation of int or float operands is float. Division of two complex numbers returns a complex number. Given below are examples of augmented division operator.
Augmented Modulus Operator (%=)
To perform modulus and assignment operation in a single statement, use the %= operator. Like the mod operator, its augmented version also is not supported for complex number.
Augmented Exponent Operator (**=)
The "**=" operator results in computation of "a" raised to "b", and assigning the value back to "a". Given below are some examples −
Augmented Floor division Operator (//=)
For performing floor division and assignment in a single statement, use the "//=" operator. "a//=b" is equivalent to "a=a//b". This operator cannot be used with complex numbers.
Assignment Expressions: The Walrus Operator
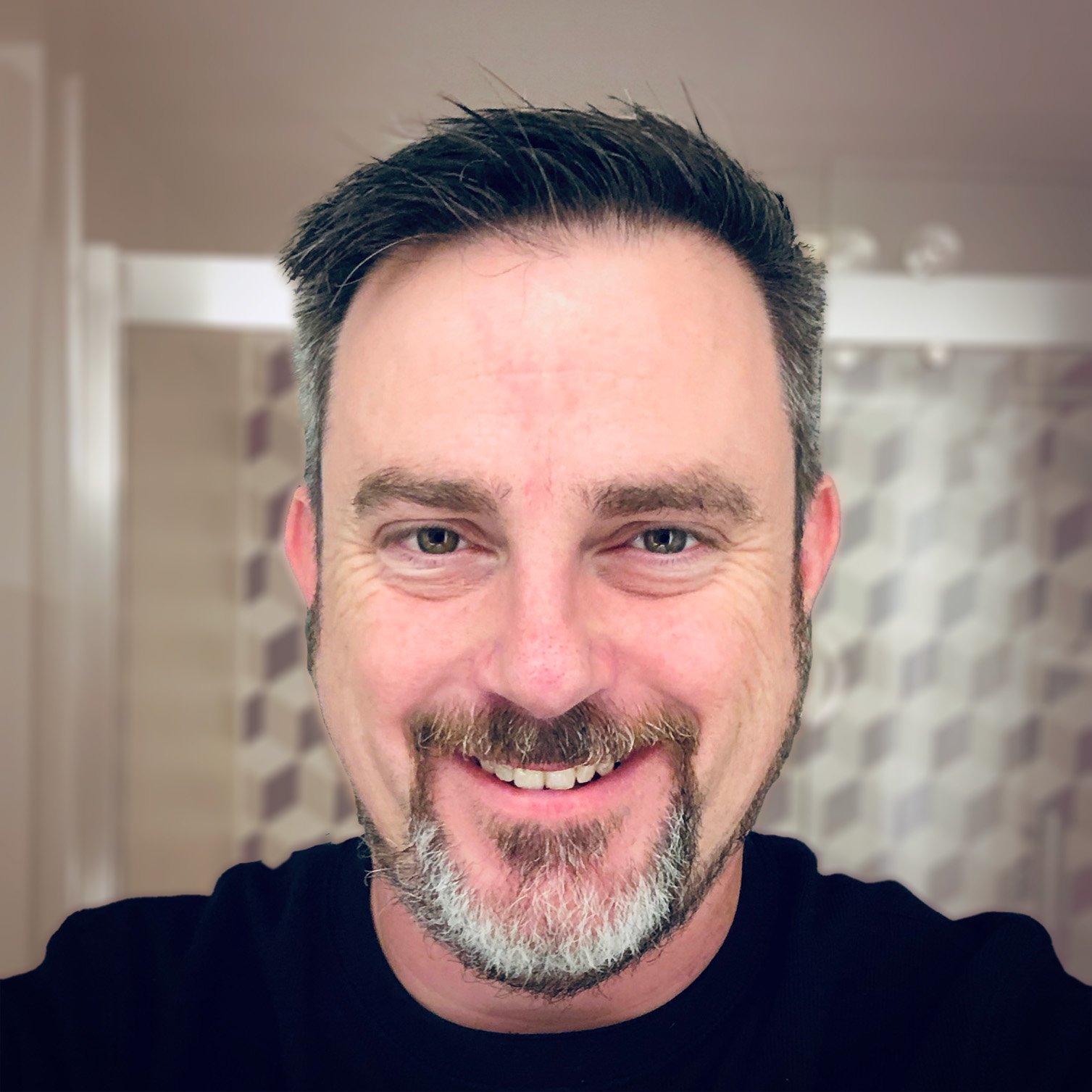
- Discussion (8)
In this lesson, you’ll learn about the biggest change in Python 3.8: the introduction of assignment expressions . Assignment expression are written with a new notation (:=) .This operator is often called the walrus operator as it resembles the eyes and tusks of a walrus on its side.
Assignment expressions allow you to assign and return a value in the same expression. For example, if you want to assign to a variable and print its value, then you typically do something like this:
In Python 3.8, you’re allowed to combine these two statements into one, using the walrus operator:
The assignment expression allows you to assign True to walrus , and immediately print the value. But keep in mind that the walrus operator does not do anything that isn’t possible without it. It only makes certain constructs more convenient, and can sometimes communicate the intent of your code more clearly.
One pattern that shows some of the strengths of the walrus operator is while loops where you need to initialize and update a variable. For example, the following code asks the user for input until they type quit :
This code is less than ideal. You’re repeating the input() statement, and somehow you need to add current to the list before asking the user for it. A better solution is to set up an infinite while loop, and use break to stop the loop:
This code is equivalent to the code above, but avoids the repetition and somehow keeps the lines in a more logical order. If you use an assignment expression, then you can simplify this loop further:
This moves the test back to the while line, where it should be. However, there are now several things happening at that line, so it takes a bit more effort to read it properly. Use your best judgement about when the walrus operator helps make your code more readable.
PEP 572 describes all the details of assignment expressions, including some of the rationale for introducing them into the language, as well as several examples of how the walrus operator can be used. The Python 3.8 documentation also includes some good examples of assignment expressions.
Here are a few resources for more info on using bpython, the REPL (Read–Eval–Print Loop) tool used in most of these videos:
- Discover bpython: A Python REPL With IDE-Like Features
- A better Python REPL: bpython vs python
- bpython Homepage
- bpython Docs
00:00 In this video, you’ll learn about what’s being called the walrus operator. One of the biggest changes in Python 3.8 is the introduction of these assignment expressions. So, what does it do?
00:12 Well, it allows the assignment and the return of a value in the same expression, using a new notation. On the left side, you’d have the name of the object that you’re assigning, and then you have the operator, a colon and an equal sign ( := ), affectionately known as the walrus operator as it resembles the eyes and tusks of a walrus on its side.
00:32 And it’s assigning this expression on the right side, so it’s assigning and returning the value in the same expression. Let me have you practice with this operator with some code.
00:44 Throughout this tutorial, when I use a REPL, I’m going to be using this custom REPL called bpython . I’ll include links on how to install bpython below this video.
00:53 So, how do you use this assignment operator? Let me have you start with a small example. You could have an object named walrus and assign it the value of False , and then you could print it. In Python 3.8, you can combine those two statements and do a single statement using the walrus operator. So inside of print() , you could say walrus , the new object, and use the operator, the assignment expression := , and a space, and then say True . That’s going to do two things. Most notably, in reverse order, it returned the value True . And then it also assigned the value to walrus , and of course the type of 'bool' .
01:38 Keep in mind, the walrus operator doesn’t do anything that isn’t possible without it. It only makes certain constructs a bit more convenient, and can sometimes communicate the intent of your code more clearly.
01:48 Let me show you another example. It’s a pattern that shows some of the strengths of the walrus operator inside of while loops, where you need to initialize and update a variable. For example, create a new file, and name it write_something.py . Here’s write_something.py .
02:09 It starts with inputs , which will be a list. So create a list called inputs .
02:16 Into an object named current , use an input() statement. The input() statement is going to provide a prompt and read a string in from standard input. The prompt will be this, "Write something: " .
02:28 So when the user inputs that, that’ll go into current . So while current != "quit" — if the person has not typed quit yet— you’re going to take inputs and append the current value.
02:44 And then here, you’re asking to "Write something: " again.
02:50 Down here at my terminal, after saving—let’s see, make sure you’re saved. Okay. Now that’s saved.
03:00 So here, I could say, Hello , Welcome , and then finally quit , which then would quit it. So, this code isn’t ideal.
03:08 You’re repeating the input() statement twice, and somehow you need to add current to the list before asking the user for it. So a better solution is going to be to set up maybe an infinite while loop, and then use a break to stop the loop. How would that look?
03:22 You’re going to rearrange this a little bit. Move the while loop up, and say while True:
03:35 and here say if current == "quit": then break . Otherwise, go ahead and append it. So, a little different here, but this is a while loop that’s going to continue as long as it doesn’t get broken out of by someone typing quit . Okay.
03:53 Running it again. And there, you can see it breaking out. Nice. So, that code avoids the repetition and kind of keeps things in a more logical order, but there’s a way to simplify this to use that new assignment expression, the walrus operator. In that case, you’re going to modify this quite a bit.
04:17 Here you’re going to say while , current and then use that assignment operator ( := ) to create current .
04:23 But also, while doing that, check to see that it’s not equal to "quit" . So here, each time that assigns the value to current and it’s returned, so the value can be checked.
04:35 So while , current , assigning the value from the input() , and then if it’s not equal to "quit" , you’re going to append current . Make sure to save.
04:42 Run the code one more time.
04:47 And it works the same. This moves that test all the way back to the while line, where it should be. However, there’s a couple of things now happening all in one line, and that might take a little more effort to read what’s happening and to understand it properly.
05:00 There are a handful of other examples that you could look into to learn a little more about assignment expressions. I’ll include a link to PEP 572, and also a link to the Python docs for version 3.8, both of which include more code examples.
05:14 So you need to use your best judgment as to when this operator’s going to make your code more readable and more useful. In the next video, you’ll learn about the new feature of positional-only arguments.
rajeshboyalla on Dec. 4, 2019
Why do you use list() to initialize a list rather than using [] ?
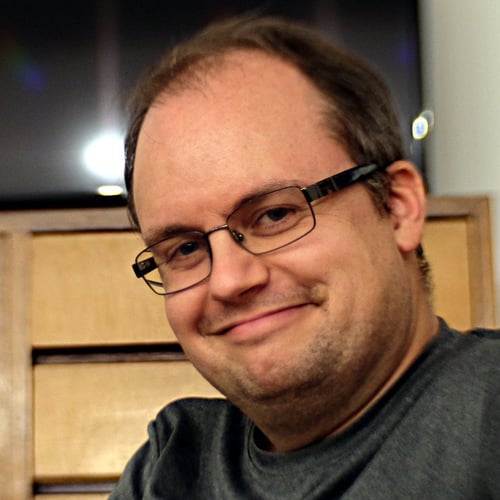
Geir Arne Hjelle RP Team on Dec. 4, 2019
My two cents about list() vs [] (I wrote the original article this video series is based on):
- I find spelling out list() to be more readable and easier to notice and interpret than []
- [] is several times faster than list() , but we’re still talking nanoseconds. On my computer [] takes about 15ns, while list() runs in 60ns. Typically, lists are initiated once, so this does not cause any meaningful slowdown of code.
That said, if I’m initializing a list with existing elements, I usually use [elem1, elem2, ...] , since list(...) has different–and sometimes surprising–semantics.
Jason on April 3, 2020
Sorry for my ignorance, did the the standard assignment = operator work in this way? I don’t understand what has been gained from adding the := operator. If anything I think it will allow people to write more obfuscated code. But minds better than mine have been working on this, so I’ll have to take their word it is an improvement.
As for the discussion on whether [] is more readable than list(). I’d never seen list() before, so to me [] is better. I’ve only just come over from the dark 2.7 side so maybe it’s an old python programmer thing?
Oh I checked the operation on the assignment operator. I was obviously wrong. lol Still I think the existing operator could’ve been tweaked to do the same thing as := … I’m still on the fence about that one.
gedece on April 3, 2020
you are right in that the existing operator could have worked, but it can lead to something unexpected.
if you do something like
if (newvar = somevar): it gives a traceback, because you are supposed to use == for comparations.
So if you use the normal operator for this, then that expression is valid and you’ll be hard pressed to realize the mistake.
It then makes complete sense to use a different operator as that helps to clarify intent in code.
Jason on April 6, 2020
Yes, I’ve accidentaly done that in other languages before and it can be a difficult to “see” bug.
varelaautumn on Sept. 26, 2020
I watched this earlier today and now tonight I just can’t stop myself from throwing these walrus operators everywhere.
(I’m new and learning so these are just personal fooling around programs)
For example I have this function which cycles through a bunch of other very simple parsing functions that check if my input string is valid in the context of the game state. If the string doesn’t pass one of these parsers it returns a string with an error message such as “Input must be less than 5 characters”. And then the parse_input function returns that error string.
I mean it’s not a huge change, but it saves an extra call of the function, and I feel like it makes it much more readable.
I’m not sure if this other case might be considered abuse of the walrus operator, but I decided to use it twice in one line.
This function repeatedly asks for input. If the input does not pass the parser functions, then the error will be returned and printed out in the while loop. Otherwise the input was valid and it gets returned.
I’m able to pass my input into a function and check the result of that function all while retaining my input and the return of the function as their own variables to be used in the next line.
I think the walrus operator helped me put all the relevant details on the three lines. Like if you just read the first words of each line, it basically says “while error, print error, else return input_string.” I don’t see how I could have done that without this cool walrus operator so I’m really appreciative for this video you made! I’ve been converted to a strong believer in the walrus operator.
Geir Arne Hjelle RP Team on Sept. 26, 2020
@varelaautumn Nice examples, thanks for sharing!
I agree that the walrus operator will not revolutionize your code, but it can bring these sorts of small improvements that add up in the long run.
Become a Member to join the conversation.
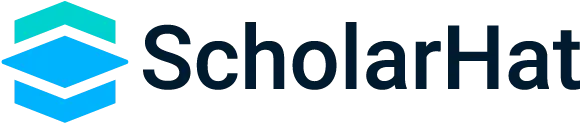
01 Career Opportunities
- 10 Python Developer Skills you must know in 2024
- Python Developer Roadmap: How to become a Python Developer?
- How to Become a Python Full Stack Developer [Step-by-Step]
- Python Career Guide: Is it worth learning in 2024?
- Top 50+ Python Interview Questions and Answers
- Python Developer Salary
02 Beginner
- Python For Loop
- Difference between For Loop and While Loop in Python
- Bitwise Operators in Python
- Logical Operators Python
- Comparison Operators Python
- Understanding Python While Loop with Examples
- Factorial Calculator in Python
- The map() Function in Python
- Calculation of Armstrong Number in Python
- Program to Check Leap Year in Python
- The enumerate() Function in Python
- How to Run a Python Script- A Step by Step Guide
- List of Python Keywords (With Examples)
- Python Program to Check Prime Number
- Lambda() Function in Python
- Fibonacci Series in Python
- Python Features: A Comprehensive Guide for Beginners
- Data Structures in Python - Types and Examples (A Complete Guide)
- Python Membership Operators: Types of Membership Operators
Assignment Operators in Python
- Arithmetic Operators in Python
- Addition of Two Numbers in Python
- What is a Python Interpreter?
- Introduction to the python language
- What is Python Language? Overview of the Python Language
- Python Basic Syntax: First Python Program
- Comments in Python: An Overview
- What are Python Variables - Types of Variables in Python Language
- Data Types in Python - 8 Data Types in Python With Examples
- What are Operators in Python - Types of Operators in Python ( With Examples )
- Decision Making Statements: If, If..else, Nested If..else and if-elif-else Ladder
- Types of Loops in Python - For, While Loop
- 10 Reasons Python is a Great First Language to Learn
- Tuples in Python with Examples - A Beginner Guide
- Python Functions: A Complete Guide
03 Intermediate
- Python Classes and Objects with Examples
- How to Use List Comprehension in Python
- OOPs Concepts in Python
- What are Strings in Python? Learn to Implement Them.
- Python Lists: List Methods and Operations
- Python Dictionaries (With Examples) : A Comprehensive Tutorial
- Modules in Python
- Exception Handling in Python: Try and Except Statement
04 Training Programs
- Java Programming Course
- MERN: Full-Stack Web Developer Certification Training
- Data Structures and Algorithms Training
- Assignment Operators In P..
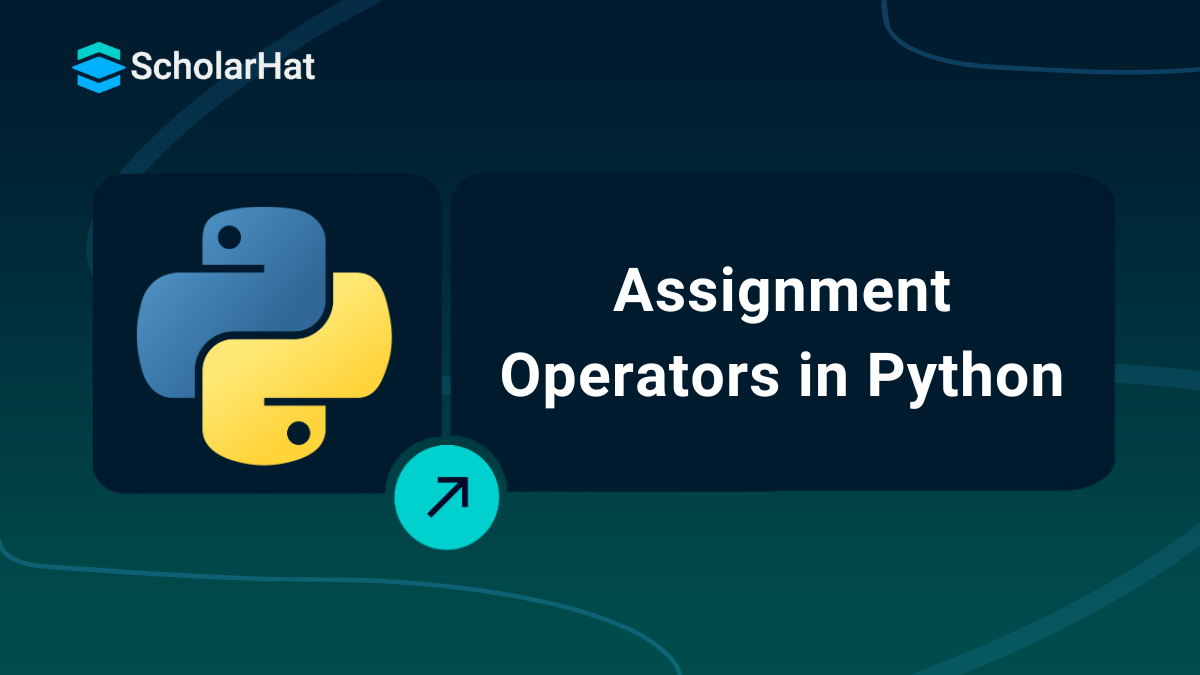
What is an Assignment Operator in Python?
Assignment Operators in Python are used to assign values to the variables. "=" is the fundamental Python assignment operator. They require two operands to operate upon. The left side operand is called a variable, and the right side operand is the value. The value on the right side of the "=" is assigned to the variable on the left side of "=". The associativity is from right to left.
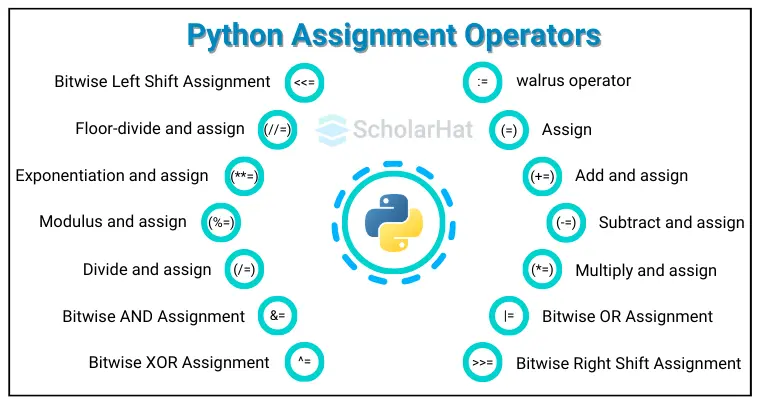

2. Augmented Assignment Operators in Python
In addition to the "=" operator, you can combine arithmetic and bitwise operators with the = symbol to form a cumulated or augmented assignment operator.
1. Augmented Arithmetic Assignment Operators in Python
There are seven combinations of arithmetic operators with the assignment operator "=." The below table gives a glimpse of them.
+= | Addition Assignment Operator |
-= | Subtraction Assignment Operator |
*= | Multiplication Assignment Operator |
/= | Division Assignment Operator |
%= | Modulus Assignment Operator |
//= | Floor Division Assignment Operator |
**= | Exponentiation Assignment Operator |
We'll see all these operators in detail in the upcoming sections.
2. Augmented Bitwise Assignment Operators in Python
There are five combinations of bitwise operators with the assignment operator "=." The below table gives a glimpse of them.
&= | Bitwise AND Assignment Operator |
|= | Bitwise OR Assignment Operator |
^= | Bitwise XOR Assignment Operator |
>>= | Bitwise Right Shift Assignment Operator |
<<= | Bitwise Left Shift Assignment Operator |
Augmented Arithmetic Assignment Operators in Python
1. augmented addition operator (+=).
It adds the right operand to the left operand and assigns the result to the left operand.
Example of Augmented Addition Operator in Python
In this example, we have assigned the value of the sum of x and y to the variable x using the assignment operator (+=).
2. Augmented Subtraction Operator (-=)
It subtracts the right operand from the left operand and assigns the result to the left operand.
Example of Augmented Subtraction Operator in Python
In this example, we have assigned the value of the difference between x and y to the variable x using the assignment operator (-=).
3. Augmented Multiplication Operator (*=)
It multiplies the right operand with the left operand and assigns the result to the left operand.
Example of Augmented Multiplication Operator in Python
In this example, we have assigned the value of the product of x and y to the variable x using the assignment operator (*=).
4. Augmented Division Operator (/=)
It divides the left operand with the right operand and assigns the result to the left operand.
Example of Augmented Division Operator in Python
In this example, we have assigned the value of the result of dividing x by y to the variable x using the assignment operator (/=).
5. Augmented Modulus Operator (%=)
It calculates the modulus of two operands and assigns the result to the left operand.
Example of Augmented Modulus Operator in Python
In this example, we have assigned the value of the modulus of x by y to the variable x using the assignment operator (%=).
6. Augmented Floor division Operator (//=)
It divides the left operand with the right operand and assigns the floor value to the left operand.
Example of Augmented Floor Division Operator in Python
In this example, we have assigned the floor value of the division of x by y to the variable x using the assignment operator (//=).
7. Augmented Exponent Operator (**=)
It calculates the exponent(raise power) value of the left operand raised to the right operand and assigns the floor value to the left operand.
Example of Augmented Exponent Operator in Python
In this example, we have assigned the exponent value of x raised to y to the variable x using the assignment operator (**=).
Augmented Bitwise Assignment Operators in Python
1. augmented bitwise and (&=).
It performs the bitwise AND operation on the left and right operands and assigns the result to the left operand.
Example of Augmented Bitwise AND Operator in Python
In this example, we have assigned the result of x & y to the variable x using the assignment operator (&=).
2. Augmented Bitwise OR (|=)
It performs the bitwise OR operation on the left and right operands and assigns the result to the left operand.
Example of Augmented Bitwise OR Operator in Python
In this example, we have assigned the result of x | y to the variable x using the assignment operator (|=).
3. Augmented Bitwise XOR (^=)
It performs the bitwise XOR operation on the left and right operands and assigns the result to the left operand.
Example of Augmented Bitwise XOR Operator in Python
In this example, we have assigned the result of x ^ y to the variable x using the assignment operator (^=).
4. Augmented Bitwise Right Shift (>>=)
It performs the bitwise right shift operation on the left and right operands and assigns the result to the left operand.
Example of Augmented Bitwise Right Shift Operator in Python
In this example, we have assigned the result of x >>= y to the variable x using the assignment operator (>>=).
5. Augmented Bitwise Left Shift (<<=)
It performs the bitwise left shift operation on the left and right operands and assigns the result to the left operand.
Example of Augmented Bitwise Left Shift Operator in Python
In this example, we have assigned the result of x <<= y to the variable x using the assignment operator (<<=).
Walrus Operator in Python
Python 3.8 introduced the walrus operator (:=) to write an assignment expression. Assignment expressions have a return value that is automatically assigned to a variable.
Syntax of an Assignment Expression
Example of walrus operator in python.
The ":=" operator assigns the value of numbers.pop(0) to the variable num and evaluates it in the Python while loop condition.
Assignment operators are critical fundamental operators that are useful for various operations. They are capable of performing assignment, bitwise, and shift operations. To learn Python effectively, you need to master these operators.
Live Classes Schedule
ASP.NET Core Certification Training | Jul 17 | MON, WED, FRI | Filling Fast | ||
Advanced Full-Stack .NET Developer Certification Training | Jul 17 | MON, WED, FRI | Filling Fast | ||
Angular Certification Course | Jul 20 | SAT, SUN | Filling Fast | ||
Generative AI For Software Developers | Jul 20 | SAT, SUN | Filling Fast | ||
Azure Master Class | Jul 20 | SAT, SUN | Filling Fast | ||
ASP.NET Core Certification Training | Jul 28 | SAT, SUN | Filling Fast | ||
Software Architecture and Design Training | Jul 28 | SAT, SUN | Filling Fast | ||
.NET Solution Architect Certification Training | Jul 28 | SAT, SUN | Filling Fast | ||
Azure Developer Certification Training | Jul 28 | SAT, SUN | Filling Fast | ||
Advanced Full-Stack .NET Developer Certification Training | Jul 28 | SAT, SUN | Filling Fast | ||
Data Structures and Algorithms Training with C# | Jul 28 | SAT, SUN | Filling Fast | ||
Angular Certification Course | Aug 11 | SAT, SUN | Filling Fast | ||
ASP.NET Core Project | Aug 24 | SAT, SUN | Filling Fast |
Can't find convenient schedule? Let us know
About Author
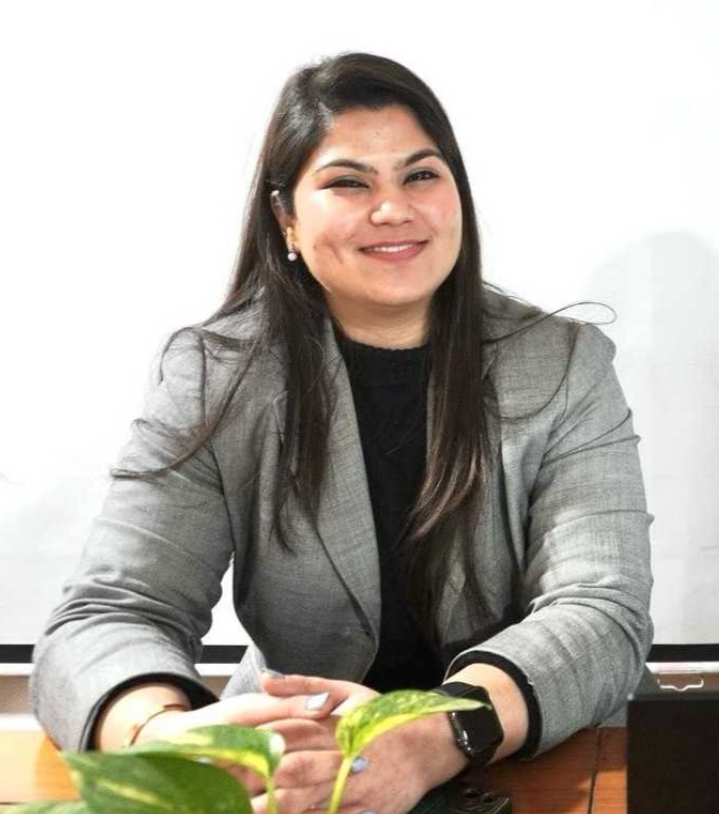
She is passionate about different technologies like JavaScript, React, HTML, CSS, Node.js etc. and likes to share knowledge with the developer community. She holds strong learning skills in keeping herself updated with the changing technologies in her area as well as other technologies like Core Java, Python and Cloud.
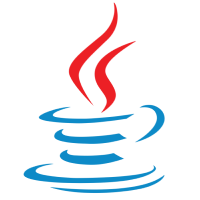
We use cookies to make interactions with our websites and services easy and meaningful. Please read our Privacy Policy for more details.
Python Operators: Arithmetic, Assignment, Comparison, Logical, Identity, Membership, Bitwise
Operators are special symbols that perform some operation on operands and returns the result. For example, 5 + 6 is an expression where + is an operator that performs arithmetic add operation on numeric left operand 5 and the right side operand 6 and returns a sum of two operands as a result.
Python includes the operator module that includes underlying methods for each operator. For example, the + operator calls the operator.add(a,b) method.
Above, expression 5 + 6 is equivalent to the expression operator.add(5, 6) and operator.__add__(5, 6) . Many function names are those used for special methods, without the double underscores (dunder methods). For backward compatibility, many of these have functions with the double underscores kept.
Python includes the following categories of operators:
Arithmetic Operators
Assignment operators, comparison operators, logical operators, identity operators, membership test operators, bitwise operators.
Arithmetic operators perform the common mathematical operation on the numeric operands.
The arithmetic operators return the type of result depends on the type of operands, as below.
- If either operand is a complex number, the result is converted to complex;
- If either operand is a floating point number, the result is converted to floating point;
- If both operands are integers, then the result is an integer and no conversion is needed.
The following table lists all the arithmetic operators in Python:
Operation | Operator | Function | Example in Python Shell |
---|---|---|---|
Sum of two operands | + | operator.add(a,b) | |
Left operand minus right operand | - | operator.sub(a,b) | |
* | operator.mul(a,b) | ||
Left operand raised to the power of right | ** | operator.pow(a,b) | |
/ | operator.truediv(a,b) | ||
equivilant to | // | operator.floordiv(a,b) | |
Reminder of | % | operator.mod(a, b) |
The assignment operators are used to assign values to variables. The following table lists all the arithmetic operators in Python:
Operator | Function | Example in Python Shell |
---|---|---|
= | ||
+= | operator.iadd(a,b) | |
-= | operator.isub(a,b) | |
*= | operator.imul(a,b) | |
/= | operator.itruediv(a,b) | |
//= | operator.ifloordiv(a,b) | |
%= | operator.imod(a, b) | |
&= | operator.iand(a, b) | |
|= | operator.ior(a, b) | |
^= | operator.ixor(a, b) | |
>>= | operator.irshift(a, b) | |
<<= | operator.ilshift(a, b) |
The comparison operators compare two operands and return a boolean either True or False. The following table lists comparison operators in Python.
Operator | Function | Description | Example in Python Shell |
---|---|---|---|
> | operator.gt(a,b) | True if the left operand is higher than the right one | |
< | operator.lt(a,b) | True if the left operand is lower than right one | |
== | operator.eq(a,b) | True if the operands are equal | |
!= | operator.ne(a,b) | True if the operands are not equal | |
>= | operator.ge(a,b) | True if the left operand is higher than or equal to the right one | |
<= | operator.le(a,b) | True if the left operand is lower than or equal to the right one |
The logical operators are used to combine two boolean expressions. The logical operations are generally applicable to all objects, and support truth tests, identity tests, and boolean operations.
Operator | Description | Example |
---|---|---|
and | True if both are true | |
or | True if at least one is true | |
not | Returns True if an expression evalutes to false and vice-versa |
The identity operators check whether the two objects have the same id value e.i. both the objects point to the same memory location.
Operator | Function | Description | Example in Python Shell |
---|---|---|---|
is | operator.is_(a,b) | True if both are true | |
is not | operator.is_not(a,b) | True if at least one is true |
The membership test operators in and not in test whether the sequence has a given item or not. For the string and bytes types, x in y is True if and only if x is a substring of y .
Operator | Function | Description | Example in Python Shell |
---|---|---|---|
in | operator.contains(a,b) | Returns True if the sequence contains the specified item else returns False. | |
not in | not operator.contains(a,b) | Returns True if the sequence does not contains the specified item, else returns False. |
Bitwise operators perform operations on binary operands.
Operator | Function | Description | Example in Python Shell |
---|---|---|---|
& | operator.and_(a,b) | Sets each bit to 1 if both bits are 1. | |
| | operator.or_(a,b) | Sets each bit to 1 if one of two bits is 1. | |
^ | operator.xor(a,b) | Sets each bit to 1 if only one of two bits is 1. | |
~ | operator.invert(a) | Inverts all the bits. | |
<< | operator.lshift(a,b) | Shift left by pushing zeros in from the right and let the leftmost bits fall off. | |
>> | operator.rshift(a,b) | Shift right by pushing copies of the leftmost bit in from the left, and let the rightmost bits fall off. |
- Compare strings in Python
- Convert file data to list
- Convert User Input to a Number
- Convert String to Datetime in Python
- How to call external commands in Python?
- How to count the occurrences of a list item?
- How to flatten list in Python?
- How to merge dictionaries in Python?
- How to pass value by reference in Python?
- Remove duplicate items from list in Python
- More Python articles
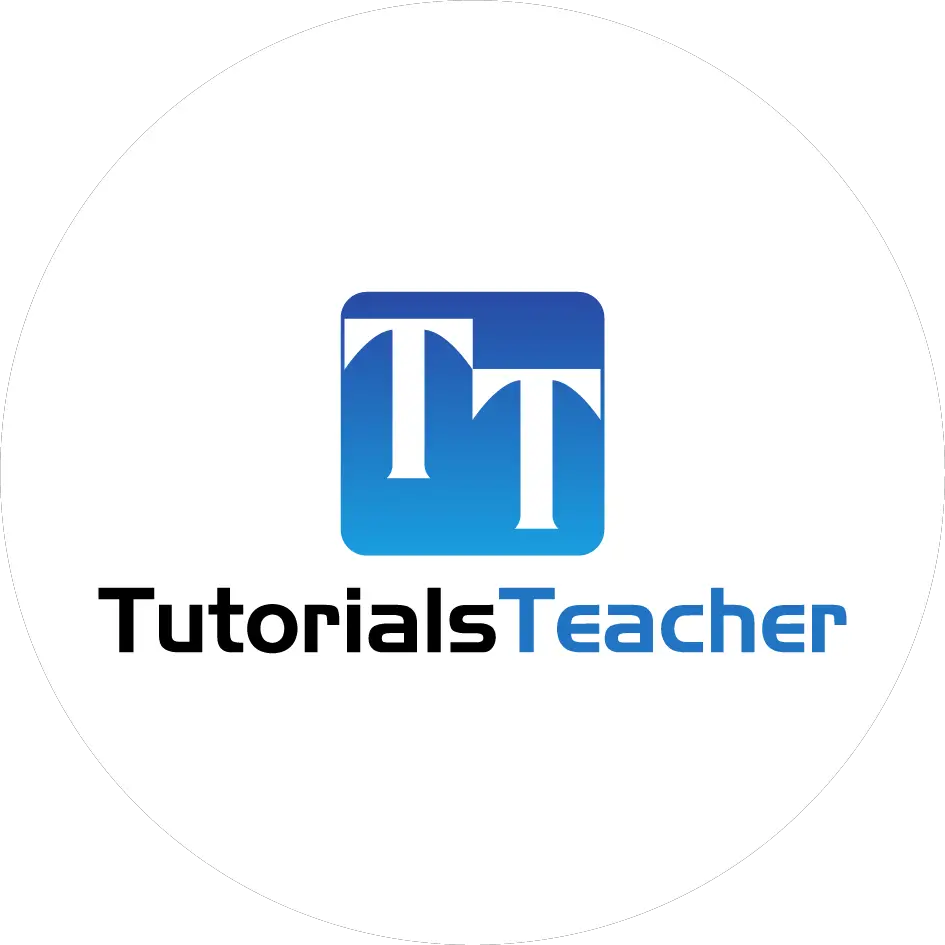
We are a team of passionate developers, educators, and technology enthusiasts who, with their combined expertise and experience, create in -depth, comprehensive, and easy to understand tutorials.We focus on a blend of theoretical explanations and practical examples to encourages hands - on learning. Visit About Us page for more information.
- Python Questions & Answers
- Python Skill Test
- Python Latest Articles
Python Assignment Operators
Lesson Contents
Python assignment operators are one of the operator types and assign values to variables . We use arithmetic operators here in combination with a variable.
Let’s take a look at some examples.
Operator Assignment (=)
This is the most basic assignment operator and we used it before in the lessons about lists , tuples , and dictionaries . For example, we can assign a value (integer) to a variable:
Operator Addition (+=)
We can add a number to our variable like this:
Using the above operator is the same as doing this:
The += operator is shorter to write but the end result is the same.
Operator Subtraction (-=)
We can also subtract a value. For example:
Using this operator is the same as doing this:
Operator Multiplication (*=)
We can also use multiplication. We’ll multiply our variable by 4:
Which is similar to:
Operator Division (/=)
Let’s try the divide operator:
This is the same as:
Operator Modulus (%=)
We can also calculate the modulus. How about this:
This is the same as doing it like this:
Operator Exponentiation (**=)
How about exponentiation? Let’s give it a try:
Which is the same as doing it like this:
Operator Floor Division (//=)
The last one, floor division:
You have now learned how to use the Python assignment operators to assign values to variables and how you can use them with arithmetic operators . I hope you enjoyed this lesson. If you have any questions, please leave a comment.
Ask a question or start a discussion by visiting our Community Forum

IMAGES
VIDEO
COMMENTS
In this tutorial, you'll learn how to use Python's assignment operators to write assignment statements that allow you to create, initialize, and update variables in your code.
An assignment operator is an operator that is used to assign some value to a variable. Like normally in Python, we write "a = 5" to assign value 5 to variable 'a'. Augmented assignment operators have a special role to play in Python programming.
Python Assignment Operators. Assignment operators are used to assign values to variables: Operator. Example. Same As. Try it. =. x = 5. x = 5.
The Walrus Operator and Assignment Expressions. Bitwise Operators and Expressions in Python. Operator Precedence in Python. Augmented Assignment Operators and Expressions. Conclusion. Remove ads. In Python, operators are special symbols, combinations of symbols, or keywords that designate some type of computation.
Python Assignment Operator. The = (equal to) symbol is defined as assignment operator in Python. The value of Python expression on its right is assigned to a single variable on its left.
Assignment expressions allow you to assign and return a value in the same expression. For example, if you want to assign to a variable and print its value, then you typically do something like this: Python. >>> walrus = False >>> print(walrus) False.
Assignment Operators in Python are used to assign values to the variables. "=" is the fundamental Python assignment operator. They require two operands to operate upon. The left side operand is called a variable, and the right side operand is the value. The value on the right side of the "=" is assigned to the variable on the left side of "=".
Assignment Operators. The assignment operators are used to assign values to variables. The following table lists all the arithmetic operators in Python:
What are Assignment Operators in Python? The assignment operators are utilized to assign values to variables. Let's take a look at each Python Assignment Operator individually now. Assign. The value of the right side of the expression is assigned to the left side operand using this operator. Syntax. x = y + z. Example. a = 13. b = 15. c = a + b.
Python assignment operators are one of the operator types and assign values to variables. We use arithmetic operators here in combination with a variable. Let’s take a look at some examples. Operator Assignment (=) This is the most basic assignment operator and we used it before in the lessons about lists, tuples, and dictionaries .