JavaScript disabled. A lot of the features of the site won't work. Find out how to turn on JavaScript HERE .

- Fundamentals
- Objects & Classes
- OO Concepts
- API Contents
- Input & Output
- Collections
- Concurrency
- Swing & RMI
- Certification

Assignment Operators J8 Home « Assignment Operators
- << Relational & Logical Operators
- Bitwise Logical Operators >>
Symbols used for mathematical and logical manipulation that are recognized by the compiler are commonly known as operators in Java. In the third of five lessons on operators we look at the assignment operators available in Java.
Assignment Operators Overview Top
The single equal sign = is used for assignment in Java and we have been using this throughout the lessons so far. This operator is fairly self explanatory and takes the form variable = expression; . A point to note here is that the type of variable must be compatible with the type of expression .
Shorthand Assignment Operators
The shorthand assignment operators allow us to write compact code that is implemented more efficiently.
Automatic Type Conversion, Assignment Rules Top
The following table shows which types can be assigned to which other types, of course we can assign to the same type so these boxes are greyed out.
When using the table use a row for the left assignment and a column for the right assignment. So in the highlighted permutations byte = int won't convert and int = byte will convert.
Casting Incompatible Types Top
The above table isn't the end of the story though as Java allows us to cast incompatible types. A cast instructs the compiler to convert one type to another enforcing an explicit type conversion.
A cast takes the form target = (target-type) expression .
There are a couple of things to consider when casting incompatible types:
- With narrowing conversions such as an int to a short there may be a loss of precision if the range of the int exceeds the range of a short as the high order bits will be removed.
- When casting a floating-point type to an integer type the fractional component is lost through truncation.
- The target-type can be the same type as the target or a narrowing conversion type.
- The boolean type is not only incompatible but also inconvertible with other types.
Lets look at some code to see how casting works and the affect it has on values:
Running the Casting class produces the following output:
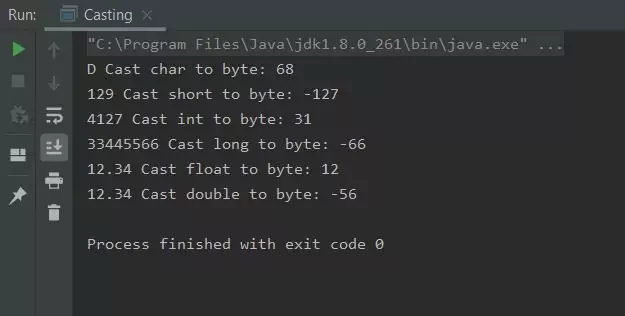
The first thing to note is we got a clean compile because of the casts, all the type conversions would fail otherwise. You might be suprised by some of the results shown in the screenshot above, for instance some of the values have become negative. Because we are truncating everything to a byte we are losing not only any fractional components and bits outside the range of a byte , but in some cases the signed bit as well. Casting can be very useful but just be aware of the implications to values when you enforce explicit type conversion.
Related Quiz
Fundamentals Quiz 8 - Assignment Operators Quiz
Lesson 9 Complete
In this lesson we looked at the assignment operators used in Java.
What's Next?
In the next lesson we look at the bitwise logical operators used in Java.
Getting Started
Code structure & syntax, java variables, primitives - boolean & char data types, primitives - numeric data types, method scope, arithmetic operators, relational & logical operators, assignment operators, assignment operators overview, automatic type conversion, casting incompatible types, bitwise logical operators, bitwise shift operators, if construct, switch construct, for construct, while construct.
Java 8 Tutorials
- Enterprise Java
- Web-based Java
- Data & Java
- Project Management
- Visual Basic
- Ruby / Rails
- Java Mobile
- Architecture & Design
- Open Source
- Web Services

Developer.com content and product recommendations are editorially independent. We may make money when you click on links to our partners. Learn More .

Java provides many types of operators to perform a variety of calculations and functions, such as logical , arithmetic , relational , and others. With so many operators to choose from, it helps to group them based on the type of functionality they provide. This programming tutorial will focus on Java’s numerous a ssignment operators.
Before we begin, however, you may want to bookmark our other tutorials on Java operators, which include:
- Arithmetic Operators
- Comparison Operators
- Conditional Operators
- Logical Operators
- Bitwise and Shift Operators
Assignment Operators in Java
As the name conveys, assignment operators are used to assign values to a variable using the following syntax:
The left side operand of the assignment operator must be a variable, whereas the right side operand of the assignment operator may be a literal value or another variable. Moreover, the value or variable on the right side must be of the same data type of the operand on the left side. Otherwise, the compiler will raise an error. Assignment operators have a right to left associativity in that the value given on the right-hand side of the operator is assigned to the variable on the left. Therefore, the right-hand side variable must be declared before assignment.
You can learn more about variables in our programming tutorial: Working with Java Variables .
Types of Assignment Operators in Java
Java assignment operators are classified into two types: simple and compound .
The Simple assignment operator is the equals ( = ) sign, which is the most straightforward of the bunch. It simply assigns the value or variable on the right to the variable on the left.
Compound operators are comprised of both an arithmetic, bitwise, or shift operator in addition to the equals ( = ) sign.
Equals Operator (=) Java Example
First, let’s learn to use the one-and-only simple assignment operator – the Equals ( = ) operator – with the help of a Java program. It includes two assignments: a literal value to num1 and the num1 variable to num2 , after which both are printed to the console to show that the values have been assigned to the numbers:
The += Operator Java Example
A compound of the + and = operators, the += adds the current value of the variable on the left to the value on the right before assigning the result to the operand on the left. Here is some sample code to demonstrate how to use the += operator in Java:
The -= Operator Java Example
Made up of the – and = operators, the -= first subtracts the variable’s value on the right from the current value of the variable on the left before assigning the result to the operand on the left. We can see it at work below in the following code example showing how to decrement in Java using the -= operator:
The *= Operator Java Example
This Java operator is comprised of the * and = operators. It operates by multiplying the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left. Here’s a program that shows the *= operator in action:
The /= Operator Java Example
A combination of the / and = operators, the /= Operator divides the current value of the variable on the left by the value on the right and then assigns the quotient to the operand on the left. Here is some example code showing how to use the /= operator in Java:
%= Operator Java Example
The %= operator includes both the % and = operators. As seen in the program below, it divides the current value of the variable on the left by the value on the right and then assigns the remainder to the operand on the left:
Compound Bitwise and Shift Operators in Java
The Bitwise and Shift Operators that we just recently covered can also be utilized in compound form as seen in the list below:
- &= – Compound bitwise Assignment operator.
- ^= – Compound bitwise ^ assignment operator.
- >>= – Compound right shift assignment operator.
- >>>= – Compound right shift filled 0 assignment operator.
- <<= – Compound left shift assignment operator.
The following program demonstrates the working of all the Compound Bitwise and Shift Operators :
Final Thoughts on Java Assignment Operators
This programming tutorial presented an overview of Java’s simple and compound assignment Operators. An essential building block to any programming language, developers would be unable to store any data in their programs without them. Though not quite as indispensable as the equals operator, compound operators are great time savers, allowing you to perform arithmetic and bitwise operations and assignment in a single line of code.
Read more Java programming tutorials and guides to software development .
Get the Free Newsletter!
Subscribe to Developer Insider for top news, trends & analysis
Latest Posts
What is the role of a project manager in software development, how to use optional in java, overview of the jad methodology, microsoft project tips and tricks, how to become a project manager in 2023, related stories, understanding types of thread synchronization errors in java, understanding memory consistency in java threads.
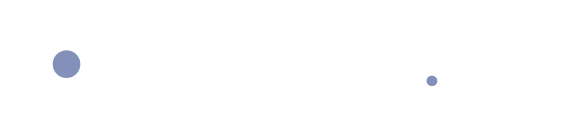
What kind of Experience do you want to share?
- Prev Class
- Next Class
- No Frames
- All Classes
- Summary:
- Nested |
- Field |
- Constr |
- Detail:
Class Boolean
- java.lang.Object
- java.lang.Boolean
Field Summary
Constructor summary, method summary, methods inherited from class java.lang. object, field detail, constructor detail, method detail, parseboolean, booleanvalue.
Submit a bug or feature For further API reference and developer documentation, see Java SE Documentation . That documentation contains more detailed, developer-targeted descriptions, with conceptual overviews, definitions of terms, workarounds, and working code examples. Copyright © 1993, 2024, Oracle and/or its affiliates. All rights reserved. Use is subject to license terms . Also see the documentation redistribution policy .
Scripting on this page tracks web page traffic, but does not change the content in any way.
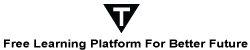
Java Tutorial
Control statements, java object class, java inheritance, java polymorphism, java abstraction, java encapsulation, java oops misc.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

Java - Compound Boolean Logical Assignment Operators
What are compound boolean logical assignment operators.
There are three compound Boolean logical assignment operators.
The operand1 must be a boolean variable and op may be &, |, or ^.
Java does not have any operators like &&= and ||=.
Compound Boolean Logical Assignment Operators are used in the form
The above form is equivalent to writing
The following table lists the compound logical assignment operators and their equivalents.
For &= operator, if both operands evaluate to true, &= returns true. Otherwise, it returns false.
For != operator, if either operand evaluates to true, != returns true. Otherwise, it returns false.
For ^= operator, if both operands evaluate to different values, that is, one of the operands is true but not both, ^= returns true. Otherwise, it returns false.

Boolean Operators
Java also supports most of the Boolean operators discussed earlier in the chapter. Let’s look at a few examples.
In Java, the not operator is the exclamation point ! , placed before a Boolean value. It will invert the value of the variable, changing true to false and false to true .
Here is a quick example:
This program will output false , which is the inverted value of b .
And &&
To perform the and operation, Java uses two ampersands && placed between Boolean values. Let’s look at an example:
This program will output true on the first line, since we know that both a and b are true . On the second line, it will output false , since c is false , even though b is true .
Similarly, Java uses two vertical pipes || to represent the or operator. On most keyboards, we can find that key above the Enter key. Here’s an example of how it can be used in code:
Once again, this program will output true on the first line, since a is true , even though b is false . On the second line, it will output false , since both b and c are false .
According to the Java documentation, Java also supports using a single & for and , and a single | for or when dealing with Boolean values. Why shouldn’t we just use these operators instead?
It turns out that there is a fundamental difference in how the Java compiler handles these operators. The double-character operators && and || are called logical operators and short-circuit operators . In some scenarios, the system only needs to look at the first value in the statement to determine the result. For example, the statement x || (y || z) will always be true if x is true , without needing to consider the values in y or z . The same works for x && (y && z) when x is false , which will result in the entire statement being false . For larger Boolean expressions, the use of these short-circuit operators can make our programs run much faster and more efficiently.
The single-character operators & and | bit-wise comparison operators and are not short-circuited. So, the system will evaluate each part of the statement before determining the outcome. For boolean values, the bit-wise operators will evaluate to the same answer as the logical operators.
However you should not use them for this purpose. First, because they will slow your programs executions. Second, because it will obscure your intent to future readers of your program. At some distance point in the future, a programmer will see you used a bit-wise operator, assume there must be a reason you did not using the logical one, and lose valuable time trying to figure out why you did not use the logical operator.
You can read more about Bitwise Operations on Wikipedia. We will not use them in this course.
Exclusive Or
Java does not support a logical exclusive or , but we can build a similar statement using other operators.
In this example, the first line will be true , since a is true but b is false . However, the second line will output false , since both a and c are true .
Comparison Operators
We can also use the comparison operators == , != , < , <= , > , and >= to compare variables containing numbers, which will result in a Boolean value. Here’s an example showing those operators in action:
As we can see, each of the comparison operators works just as we’d expect, and outputs a Boolean value of either true or false , depending on the result of the comparison.
Like most high level languages, Java does not allow the chaining of comparison operators. 10 <= x <=20 , which is a pretty standard math notation for x is between 10 and 20, will not work. First the compiler will evaluate 10 <=x as a boolean, then it will throw a fit about trying to compare a boolean and and int for the <= 20 part.
You will need to write this as 10 <= x && x <= 20 .
Order of Operations
Now that we’ve introduced some additional operators, we should also see where they fit in with the other operators in Java. Here is an updated list giving the appropriate operator precedence for Java, with new entries in bold :
- Parentheses
- Postfix: Increment ++ & Decrement -- after variable*
- Prefix: Inverse - , Not ! , Increment ++ & Decrement -- before variable*
- Multiplicative : Multiplication * , Division / , and Modulo %
- Additive : Addition + , Subtraction -
- Relational : < , > , <= , >=
- Equality : == , !=
- Logical And : &&
- Logical Or : ||
- Assignment : = , += , -= , *= , /= , %=
Last modified by: Russell Feldhausen Jul 6, 2023
Java Tutorial
Java methods, java classes, java file handling, java how to's, java reference, java examples, java booleans.
Very often, in programming, you will need a data type that can only have one of two values, like:
- TRUE / FALSE
For this, Java has a boolean data type, which can store true or false values.
Boolean Values
A boolean type is declared with the boolean keyword and can only take the values true or false :
Try it Yourself »
However, it is more common to return boolean values from boolean expressions, for conditional testing (see below).
Boolean Expression
A Boolean expression returns a boolean value: true or false .
This is useful to build logic, and find answers.
For example, you can use a comparison operator , such as the greater than ( > ) operator, to find out if an expression (or a variable) is true or false:
Or even easier:
In the examples below, we use the equal to ( == ) operator to evaluate an expression:
Real Life Example
Let's think of a "real life example" where we need to find out if a person is old enough to vote.
In the example below, we use the >= comparison operator to find out if the age ( 25 ) is greater than OR equal to the voting age limit, which is set to 18 :
Cool, right? An even better approach (since we are on a roll now), would be to wrap the code above in an if...else statement, so we can perform different actions depending on the result:
Output "Old enough to vote!" if myAge is greater than or equal to 18 . Otherwise output "Not old enough to vote.":
Booleans are the basis for all Java comparisons and conditions.
You will learn more about conditions ( if...else ) in the next chapter.
Test Yourself With Exercises
Fill in the missing parts to print the values true and false :
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
boolean assignment

Java API Documentation The Java Tutorial
7 Best Java Homework Help Websites: How to Choose Your Perfect Match?
J ava programming is not a field that could be comprehended that easily; thus, it is no surprise that young learners are in search of programming experts to get help with Java homework and handle their assignments. But how to choose the best alternative when the number of proposals is enormous?
In this article, we are going to talk about the top ‘do my Java assignment’ services that offer Java assignment assistance and dwell upon their features. In the end, based on the results, you will be able to choose the one that meets your demands to the fullest and answer your needs. Here is the list of services that are available today, as well as those that are on everyone's lips:
TOP Java Assignment Help Services: What Makes Them Special?
No need to say that every person is an individual and the thing that suits a particular person could not meet the requirements of another. So, how can we name the best Java assignment help services on the web? - We have collected the top issues students face when searching for Java homework help and found the companies that promise to meet these requirements.
What are these issues, though?
- Pricing . Students are always pressed for budget, and finding services that suit their pockets is vital. Thus, we tried to provide services that are relatively affordable on the market. Of course, professional services can’t be offered too cheaply, so we have chosen the ones that balance professionalism and affordability.
- Programming languages . Not all companies have experts in all possible programming languages. Thus, we tried to choose the ones that offer as many different languages as possible.
- Expert staff . In most cases, students come to a company when they need to place their ‘do my Java homework’ orders ASAP. Thus, a large expert staff is a real benefit for young learners. They want to come to a service, place their order and get a professional to start working on their project in no time.
- Reviews . Of course, everyone wants to get professional help with Java homework from a reputable company that has already completed hundreds of Java assignments for their clients. Thus, we have mentioned only those companies that have earned enough positive feedback from their clients.
- Deadline options. Flexible deadline options are also a benefit for those who are placing their last-minute Java homework help assignments. Well, we also provide services with the most extended deadlines for those who want to save some money and place their projects beforehand.
- Guarantees . This is the must-feature if you want to get quality assistance and stay assured you are totally safe with the company you have chosen. In our list, we have only named companies that provide client-oriented guarantees and always keep their word, as well as offer only professional Java assignment experts.
- Customization . Every service from the list offers Java assistance tailored to clients’ personal needs. There, you won’t find companies that offer pre-completed projects and sell them at half-price.
So, let’s have a closer look at each option so you can choose the one that totally meets your needs.
DoMyAssignments.com
At company service, you can get assistance with academic writing as well as STEM projects. The languages you can get help with are C#, C++, Computer science, Java, Javascript, HTML, PHP, Python, Ruby, and SQL.
The company’s prices start at $30/page for a project that needs to be done in 14+ days.
Guarantees and extra services
The company offers a list of guarantees to make your cooperation as comfortable as possible. So, what can you expect from the service?
- Free revisions . When you get your order, you can ask your expert for revisions if needed. It means that if you see that any of your demands were missed, you can get revisions absolutely for free.
- Money-back guarantee. The company offers professional help, and they are sure about their experts and the quality of their assistance. Still, if you receive a project that does not meet your needs, you can ask for a full refund.
- Confidentiality guarantee . Stay assured that all your personal information is safe and secure, as the company scripts all the information you share with them.
- 100% customized assistance . At this service, you won’t find pre-written codes, all the projects are completed from scratch.
Expert staff
If you want to hire one of the top Java homework experts at DoMyAssignments , you can have a look at their profile, see the latest orders they have completed, and make sure they are the best match for your needs. Also, you can have a look at the samples presented on their website and see how professional their experts are. If you want to hire a professional who completed a particular sample project, you can also turn to a support team and ask if you can fire this expert.
CodingHomeworkHelp.org
CodingHomeworkHelp is rated at 9.61/10 and has 10+ years of experience in the programming assisting field. Here, you can get help with the following coding assignments: MatLab, Computer Science, Java, HTML, C++, Python, R Studio, PHP, JavaScript, and C#.
Free options all clients get
Ordering your project with CodingHomeworkHelp.org, you are to enjoy some other options that will definitely satisfy you.
- Partial payments . If you order a large project, you can pay for it in two parts. Order the first one, get it done, and only then pay for the second one.
- Revisions . As soon as you get your order, you can ask for endless revisions unless your project meets your initial requirements.
- Chat with your expert . When you place your order, you get an opportunity to chat directly with your coding helper. If you have any questions or demands, there is no need to first contact the support team and ask them to contact you to your assistant.
- Code comments . If you have questions concerning your code, you can ask your helper to provide you with the comments that will help you better understand it and be ready to discuss your project with your professor.
The prices start at $20/page if you set a 10+ days deadline. But, with CodingHomeworkHelp.org, you can get a special discount; you can take 20% off your project when registering on the website. That is a really beneficial option that everyone can use.
CWAssignments.com
CWAssignments.com is an assignment helper where you can get professional help with programming and calculations starting at $30/page. Moreover, you can get 20% off your first order.
Working with the company, you are in the right hands and can stay assured that the final draft will definitely be tailored to your needs. How do CWAssignments guarantee their proficiency?
- Money-back guarantee . If you are not satisfied with the final work, if it does not meet your expectations, you can request a refund.
- Privacy policy . The service collects only the data essential to complete your order to make your cooperation effective and legal.
- Security payment system . All the transactions are safe and encrypted to make your personal information secure.
- No AI-generated content . The company does not use any AI tools to complete their orders. When you get your order, you can even ask for the AI detection report to see that your assignment is pure.
With CWAssignments , you can regulate the final cost of your project. As it was mentioned earlier, the prices start at $30/page, but if you set a long-term deadline or ask for help with a Java assignment or with a part of your task, you can save a tidy sum.
DoMyCoding.com
This company has been offering its services on the market for 18+ years and provides assistance with 30+ programming languages, among which are Python, Java, C / C++ / C#, JavaScript, HTML, SQL, etc. Moreover, here, you can get assistance not only with programming but also with calculations.
Pricing and deadlines
With DoMyCoding , you can get help with Java assignments in 8 hours, and their prices start at $30/page with a 14-day deadline.
Guarantees and extra benefits
The service offers a number of guarantees that protect you from getting assistance that does not meet your requirements. Among the guarantees, you can find:
- The money-back guarantee . If your order does not meet your requirements, you will get a full refund of your order.
- Free edits within 7 days . After you get your project, you can request any changes within the 7-day term.
- Payments in parts . If you have a large order, you can pay for it in installments. In this case, you get a part of your order, check if it suits your needs, and then pay for the other part.
- 24/7 support . The service operates 24/7 to answer your questions as well as start working on your projects. Do not hesitate to use this option if you need to place an ASAP order.
- Confidentiality guarantee . The company uses the most secure means to get your payments and protects the personal information you share on the website to the fullest.
More benefits
Here, we also want to pay your attention to the ‘Samples’ section on the website. If you are wondering if a company can handle your assignment or you simply want to make sure they are professionals, have a look at their samples and get answers to your questions.
AssignCode.com
AssignCode is one of the best Java assignment help services that you can entrust with programming, mathematics, biology, engineering, physics, and chemistry. A large professional staff makes this service available to everyone who needs help with one of these disciplines. As with some of the previous companies, AssignCode.com has reviews on different platforms (Reviews.io and Sitejabber) that can help you make your choice.
As with all the reputed services, AssignCode offers guarantees that make their cooperation with clients trustworthy and comfortable. Thus, the company guarantees your satisfaction, confidentiality, client-oriented attitude, and authenticity.
Special offers
Although the company does not offer special prices on an ongoing basis, regular clients can benefit from coupons the service sends them via email. Thus, if you have already worked with the company, make sure to check your email before placing a new one; maybe you have received a special offer that will help you save some cash.
AssignmentShark.com
Reviews about this company you can see on different platforms. Among them are Reviews.io (4.9 out of 5), Sitejabber (4.5 points), and, of course, their own website (9.6 out of 10). The rate of the website speaks for itself.
Pricing
When you place your ‘do my Java homework’ request with AssignmentShark , you are to pay $20/page for the project that needs to be done in at least ten days. Of course, if the due date is closer, the cost will differ. All the prices are presented on the website so that you can come, input all the needed information, and get an approximate calculation.
Professional staff
On the ‘Our experts’ page, you can see the full list of experts. Or, you can use filters to see the professional in the required field.
The company has a quick form on its website for those who want to join their professional staff, which means that they are always in search of new experts to make sure they can provide clients with assistance as soon as the need arises.
Moreover, if one wants to make sure the company offers professional assistance, one can have a look at the latest orders and see how experts provide solutions to clients’ orders.
What do clients get?
Placing orders with the company, one gets a list of inclusive services:
- Free revisions. You can ask for endless revisions until your order fully meets your demands.
- Code comments . Ask your professional to provide comments on the codes in order to understand your project perfectly.
- Source files . If you need the list of references and source files your helper turned to, just ask them to add these to the project.
- Chat with the professional. All the issues can be solved directly with your coding assistant.
- Payment in parts. Large projects can be paid for in parts. When placing your order, let your manager know that you want to pay in parts.
ProgrammingDoer.com
ProgrammingDoer is one more service that offers Java programming help to young learners and has earned a good reputation among previous clients.
The company cherishes its reputation and does its best to let everyone know about their proficiency. Thus, you, as a client, can read what people think about the company on several platforms - on their website as well as at Reviews.io.
What do you get with the company?
Let’s have a look at the list of services the company offers in order to make your cooperation with them as comfortable as possible.
- Free revisions . If you have any comments concerning the final draft, you can ask your professional to revise it for free as many times as needed unless it meets your requirements to the fullest.
- 24/7 assistance . No matter when you realize that you have a programming assignment that should be done in a few days. With ProgrammingDoer, you can place your order 24/7 and get a professional helper as soon as there is an available one.
- Chat with the experts . When you place your order with the company, you get an opportunity to communicate with your coding helper directly to solve all the problems ASAP.
Extra benefits
If you are not sure if the company can handle your assignment the right way, if they have already worked on similar tasks, or if they have an expert in the needed field, you can check this information on your own. First, you can browse the latest orders and see if there is something close to the issue you have. Then, you can have a look at experts’ profiles and see if there is anyone capable of solving similar issues.
Can I hire someone to do my Java homework?
If you are not sure about your Java programming skills, you can always ask a professional coder to help you out. All you need is to find the service that meets your expectations and place your ‘do my Java assignment’ order with them.
What is the typical turnaround time for completing a Java homework assignment?
It depends on the service that offers such assistance as well as on your requirements. Some companies can deliver your project in a few hours, but some may need more time. But, you should mind that fast delivery is more likely to cost you some extra money.
What is the average pricing structure for Java assignment help?
The cost of the help with Java homework basically depends on the following factors: the deadline you set, the complexity level of the assignment, the expert you choose, and the requirements you provide.
How will we communicate and collaborate on my Java homework?
Nowadays, Java assignment help companies provide several ways of communication. In most cases, you can contact your expert via live chat on a company’s website, via email, or a messenger. To see the options, just visit the chosen company’s website and see what they offer.
Regarding the Author:
Nayeli Ellen, a dynamic editor at AcademicHelp, combines her zeal for writing with keen analytical skills. In her comprehensive review titled " Programming Assignment Help: 41 Coding Homework Help Websites ," Nayeli offers an in-depth analysis of numerous online coding homework assistance platforms.

IMAGES
VIDEO
COMMENTS
The |= is a compound assignment operator ( JLS 15.26.2) for the boolean logical operator | ( JLS 15.22.2 ); not to be confused with the conditional-or || ( JLS 15.24 ). There are also &= and ^= corresponding to the compound assignment version of the boolean logical & and ^ respectively. In other words, for boolean b1, b2, these two are ...
a |= b; is the same as. a = (a | b); It calculates the bitwise OR of the two operands, and assigns the result to the left operand. To explain your example code: for (String search : textSearch.getValue()) matches |= field.contains(search); I presume matches is a boolean; this means that the bitwise operators behave the same as logical operators.
variable operator value; Types of Assignment Operators in Java. The Assignment Operator is generally of two types. They are: 1. Simple Assignment Operator: The Simple Assignment Operator is used with the "=" sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
Learning the operators of the Java programming language is a good place to start. Operators are special symbols that perform specific operations on one, two, or three operands, and then return a result. As we explore the operators of the Java programming language, it may be helpful for you to know ahead of time which operators have the highest ...
This beginner Java tutorial describes fundamentals of programming in the Java programming language ... You can also combine the arithmetic operators with the simple assignment operator to create compound assignments. For ... negating an expression, or inverting the value of a boolean. Operator Description + Unary plus operator; indicates ...
We can use it to invert the value of a boolean variable or value: boolean aTrue = true; boolean bFalse = !aTrue; 3.4. The Increment Operator ... Next, let's see which assignment operators we can use in Java. 9.1. The Simple Assignment Operator. The simple assignment operator (=) is a straightforward but important operator in Java. Actually ...
Compound Assignment Operators. An assignment operator is a binary operator that assigns the result of the right-hand side to the variable on the left-hand side. The simplest is the "=" assignment operator: int x = 5; This statement declares a new variable x, assigns x the value of 5 and returns 5. Compound Assignment Operators are a shorter ...
Assignment Operators Overview Top. The single equal sign = is used for assignment in Java and we have been using this throughout the lessons so far. This operator is fairly self explanatory and takes the form variable = expression; . A point to note here is that the type of variable must be compatible with the type of expression.
Java assignment operators are classified into two types: simple and compound. The Simple assignment operator is the equals ( =) sign, which is the most straightforward of the bunch. It simply assigns the value or variable on the right to the variable on the left. Compound operators are comprised of both an arithmetic, bitwise, or shift operator ...
The following are all possible assignment operator in java: 1. += (compound addition assignment operator) 2. -= (compound subtraction assignment operator) 3. *= (compound multiplication assignment operator) 4. /= (compound division assignment operator) 5. %= (compound modulo assignment operator)
Java Comparison Operators. Comparison operators are used to compare two values (or variables). This is important in programming, because it helps us to find answers and make decisions. The return value of a comparison is either true or false. These values are known as Boolean values, and you will learn more about them in the Booleans and If ...
The Boolean class wraps a value of the primitive type boolean in an object. An object of type Boolean contains a single field whose type is boolean . In addition, this class provides many methods for converting a boolean to a String and a String to a boolean, as well as other constants and methods useful when dealing with a boolean.
To assign a value to a variable, use the basic assignment operator (=). It is the most fundamental assignment operator in Java. It assigns the value on the right side of the operator to the variable on the left side. Example: int x = 10; int x = 10; In the above example, the variable x is assigned the value 10.
Java also supports a number of Boolean, string, and assignment operators. Boolean operators are used to perform logical comparisons, and always result in one of two values: true or false. Following are the most commonly used Boolean operators :
There are three compound Boolean logical assignment operators. The operand1 must be a boolean variable and op may be &, |, or ^. Java does not have any operators like &&= and ||=. Compound Boolean Logical Assignment Operators are used in the form. The above form is equivalent to writing. The following table lists the compound logical assignment ...
Boolean Operators. Java also supports most of the Boolean operators discussed earlier in the chapter. Let's look at a few examples. Not !. In Java, the not operator is the exclamation point !, placed before a Boolean value.It will invert the value of the variable, changing true to false and false to true.. Here is a quick example:
Java Booleans. Very often, in programming, you will need a data type that can only have one of two values, like: YES / NO. ON / OFF. TRUE / FALSE. For this, Java has a boolean data type, which can store true or false values.
Boolean value assignment in Java. 28. Java boolean |= operator. 43. How Does The Bitwise & (AND) Work In Java? 2. Need help understanding this line. 3. Bitwise and (&) operator. 0. Unable to understand Bitwise & operator in java. Hot Network Questions Travelling to Iceland via Germany with Germany-issued Schengen visa
Hi, once again I am befuddled by Java. The issue is performing an assignment for a boolean variable within a if statement: The issue is performing an assignment for a boolean variable within a if statement:
The assignment-operator version avoids temporaries and may have produced more efficient code on early non-optimising compilers. ... (a boolean in java); there is no single-bit type in C and the logical operators there apply to all scalar types. - p00ya. Feb 24, 2010 at 9:29. before C99, C didn't even have a bool type. That's 20 years in the ...
AssignCode. is one of the best Java assignment help services that you can entrust with programming, mathematics, biology, engineering, physics, and chemistry. A large professional staff makes this ...
4. You've got it right. The operator precedence rules make sure that first the == operator is evaluated. That's b1==false, yielding true. After that, the assigned is executed, setting b2 to true. Finally, the assignment operator returns the value as b2, which is evaluated by the if statement. Java usually evaluates the terms from the left to ...